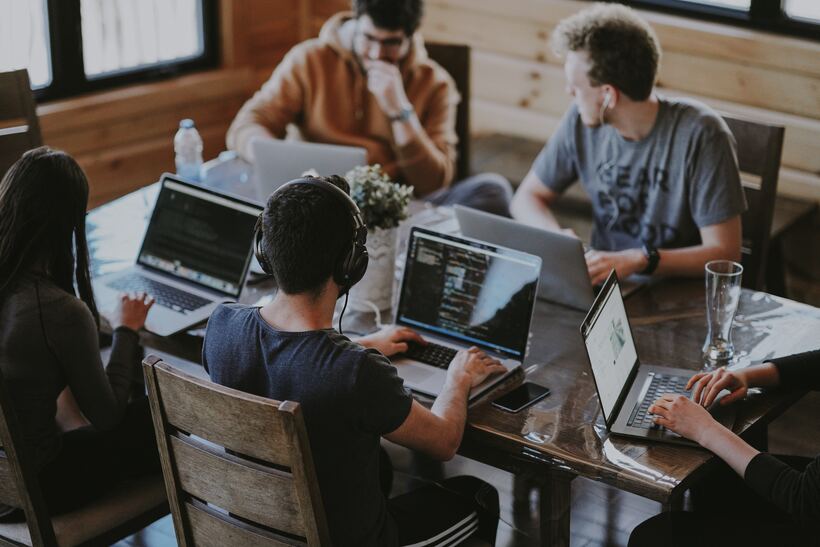
Welcome to the world of Laravel, where scalability meets elegance! If you’re a developer seeking a framework that not only simplifies your coding process but also offers robust scalability solutions, then you’ve come to the right place. In this blog post, we will dive deep into Laravel’s Event System and explore how it can take your applications to new heights of scalability.
Laravel’s Event System is like a magic wand in the hands of developers. It allows you to decouple different components of your application and build scalable architectures with ease. By leveraging events, you can make your code more maintainable, flexible, and adaptable to future changes. So let’s buckle up and unravel the secrets behind Laravel’s powerful event-driven architecture!
Benefits of Using Events for Scalability
Using events in a Laravel project can bring numerous benefits when it comes to scalability. One of the key advantages is decoupling components, allowing for a more flexible and modular architecture. By using events, you can ensure that different parts of your application are loosely coupled, meaning changes in one area won’t have a ripple effect throughout the entire codebase.
With decoupled components, you can easily add or remove functionality without impacting other parts of the system. This makes it much easier to scale your application as new features or modules can be added independently.
Another benefit of using events for scalability is improved performance. Instead of tightly coupling various processes together, you can distribute workloads across multiple event listeners. This allows for better utilization of resources and helps prevent bottlenecks during high traffic periods.
Events also enable asynchronous processing, which further enhances scalability by reducing response times. For example, if you have a task that takes some time to complete (such as sending emails), instead of blocking the main execution flow until it finishes, you can dispatch an event and let it be handled asynchronously.
Leveraging events promotes reusability within your codebase. By utilizing well-defined events and listeners, you create building blocks that can be easily reused across different parts of your application or even in future projects.
In conclusion: Using Laravel’s event system provides several benefits when it comes to scalability in web applications. It enables decoupling between components, improves performance through distributed workloads and asynchronous processing while promoting reusability within the codebase.
How Events Work in Laravel
Laravel’s event system is a powerful tool that allows developers to decouple different components of an application and improve its scalability. But how exactly do events work in Laravel?
At the core of Laravel’s event system is the concept of “event listeners.” These listeners are responsible for handling specific events that occur within the application. When an event is fired, all registered listeners for that event will be executed.
To understand this better, let’s consider an example. Imagine you have a user registration form on your website. After a successful registration, you want to send a welcome email to the new user. Instead of directly sending the email right after registration, you can use Laravel’s event system to fire an “UserRegistered” event.
Once this event is fired, you can define an event listener that listens for this specific event and sends the welcome email when it occurs. This way, any additional actions or processes related to user registration can be easily added by simply registering more listeners for the same “UserRegistered”event.
By using events in Laravel, developers can achieve loose coupling between different parts of their applications and make them more flexible and scalable. Events allow multiple functionalities to be triggered simultaneously without tightly coupling them together.
In addition to providing flexibility and scalability, Laravel’s events also facilitate code organization and maintainability. With events, it becomes easier to locate relevant pieces of code as they are logically grouped based on their respective events.
Understanding how events work in Laravel opens up endless possibilities when it comes to building scalable applications with clean code architecture. It allows developers to modularize their applications effectively while keeping different components loosely coupled yet highly efficient.
Customizing and Handling Events in Laravel
Customizing and handling events in Laravel is a powerful feature that allows developers to tailor the event system according to their project’s specific needs. With Laravel, you have complete control over how events are triggered, handled, and even modified.
To customize an event in Laravel, you can create a custom event class that extends the base `Event` class provided by Laravel. This gives you the flexibility to add additional properties or methods to the event object, making it easier to pass data between different parts of your application. You can also define any necessary logic within the custom event class itself.
Handling events in Laravel is straightforward and intuitive. By creating listeners for specific events, you can define what actions should be taken when those events are fired. Listeners can be registered using simple configuration files or through anonymous functions directly in your code.
One of the great things about handling events in Laravel is that it provides multiple ways of listening for events. You can listen for an event globally across your entire application or only within certain namespaces or groups of classes. This level of granularity ensures that your code remains organized and easy to maintain.
Laravel also offers support for queuing events, which is particularly useful when dealing with time-consuming tasks or processes that don’t need immediate attention. By simply adding a `ShouldQueue` interface to your listener class, you can instruct Laravel to handle the event asynchronously using its built-in queueing system.
Additionally, you have the option to prioritize certain listeners over others by specifying their order of execution. This comes in handy when there are dependencies between listeners or when you need precise control over how different parts of your application respond to an event.
Customizing and handling events in Laravel gives developers immense flexibility and control over their applications’ behavior. Whether it’s extending default functionality with customizations or organizing complex workflows with queued listeners – Laravels’ robust event system has got developers covered! So go ahead and leverage the power of events to create scalable and maintainable Laravel projects.
Implementing Event-Driven Architecture with Laravel
Implementing Event-Driven Architecture with Laravel is a powerful way to build scalable and flexible applications. By leveraging the event system provided by Laravel, developers can design their codebase in a decoupled manner, making it easier to maintain and extend.
In an event-driven architecture, events are used as the primary means of communication between different components of the application. Instead of tightly coupling components together, events act as messages that trigger actions or updates across the system. This promotes loose coupling and allows for easier integration of new features or modifications without impacting existing functionality.
With Laravel’s event system, you can define custom events and listeners to handle specific scenarios within your application. Events represent important occurrences or changes in state while listeners are responsible for executing logic in response to those events. This separation of concerns ensures that each component only focuses on its specific task, leading to more manageable codebases.
To implement event-driven architecture with Laravel, start by defining your custom events using the `event` command-line tool provided by the framework. Then create listeners that will respond to these events by performing certain actions such as sending emails or updating database records.
By utilizing this approach, you can easily add new features or modify existing ones without having to extensively modify your core codebase. For example, imagine adding a new payment gateway integration: instead of modifying various parts of your application directly related to payments, you could simply create a listener for a “payment successful” event and handle all necessary logic there.
Many real-life examples demonstrate how effective Laravel’s event-driven architecture can be for building scalable applications. E-commerce platforms often use this approach when handling order processing or inventory management tasks asynchronously through events and listeners.
Implementing an event-driven architecture with Laravel empowers developers with a flexible and scalable solution for building complex applications. By leveraging custom events and listeners effectively, developers can ensure better maintainability while allowing easy extensibility without disrupting existing functionality.
Real-life Examples of Scalable Applications using Laravel’s Event System
1. E-commerce Platforms: One common example of a scalable application that utilizes Laravel’s event system is e-commerce platforms. These platforms handle a large number of users, products, and transactions simultaneously. By leveraging events, developers can easily trigger actions such as sending email notifications to customers when their order status changes or updating inventory levels in real-time.
2. Social Networking Sites: Another real-life example where Laravel’s event system shines is social networking sites like Facebook or Twitter. These platforms handle millions of user interactions every second, including likes, comments, and messages. With the help of events, developers can efficiently manage these interactions by triggering specific actions based on user activities.
3. Real-Time Chat Applications: Real-time chat applications also benefit greatly from Laravel’s event system. Whether it’s a messaging app for businesses or a customer support chat feature on an e-commerce platform, events allow seamless communication between users with instant updates on new messages received and sent.
4. Job Boards and Recruitment Portals: Job boards and recruitment portals require scalability to handle numerous job listings and candidate profiles while providing timely notifications to both employers and candidates about new job opportunities or application updates. By utilizing Laravel’s event system effectively, these platforms can streamline their processes while ensuring efficient data management.
5. Collaboration Tools: Collaboration tools like project management software often involve multiple users working together on various tasks simultaneously. Events play a crucial role in keeping everyone updated about changes made by team members so that collaboration remains seamless without any information gaps.
Laravel’s event system plays a vital role in ensuring scalability by allowing developers to decouple components within the application architecture and respond to specific triggers efficiently.
Leveraging the Power of Events for Scalability in Laravel Projects
The event system in Laravel is a powerful tool that allows developers to create scalable applications with ease. By implementing events, you can decouple components, improve code organization, and enhance the maintainability of your codebase.
Events provide a flexible way to handle complex workflows and ensure that different parts of your application can react to specific actions or changes. With Laravel’s event system, you have full control over how events are customized and handled within your project.
By adopting an event-driven architecture in Laravel, you can build highly scalable applications that can easily handle increased traffic or workload without sacrificing performance. The ability to asynchronously process events enables your application to respond quickly and efficiently even under heavy loads.
Real-life examples have shown us just how effective Laravel’s event system is when it comes to scalability. From e-commerce platforms handling thousands of concurrent transactions to social media networks processing millions of user interactions, using events has proven vital in ensuring smooth operations and seamless user experiences.
Understanding and leveraging the power of events in Laravel projects is essential for creating scalable applications that can grow alongside your business needs. So don’t hesitate – dive into the world of events in Laravel today and unlock new possibilities for scalability!