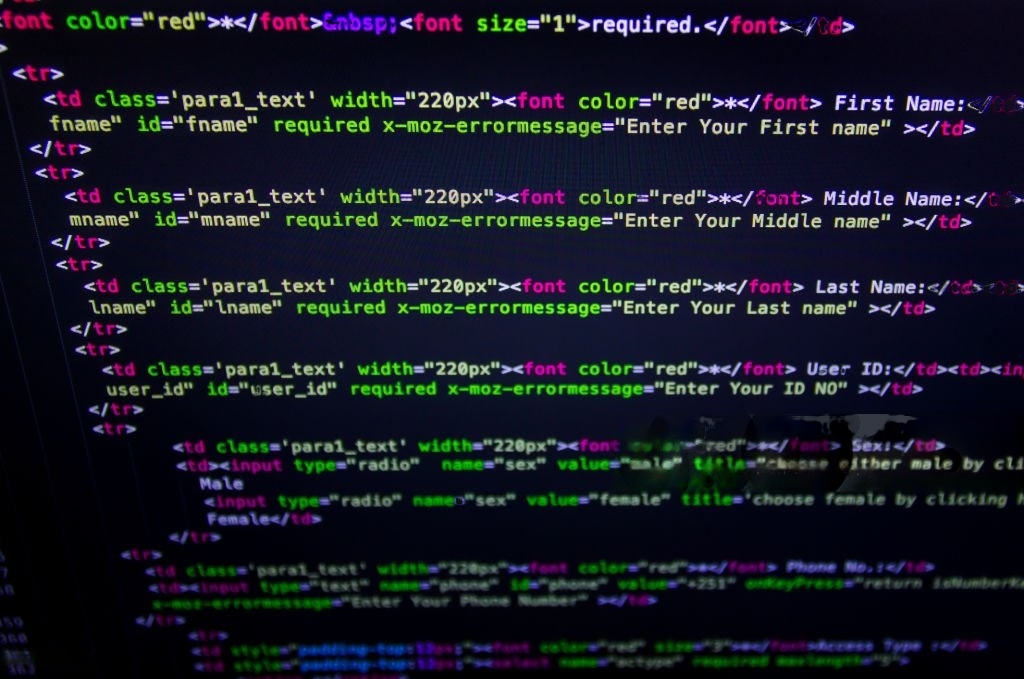
Are you ready to unlock the power of Laravel and take your API development skills to new heights? Look no further, because in this blog post, we will delve into the art of building scalable APIs in Laravel. Whether you’re a seasoned developer or just starting out on your coding journey, understanding how to create efficient and powerful APIs is essential in today’s digital landscape.
APIs, or Application Programming Interfaces, have become the backbone of modern software development. They act as intermediaries that allow different applications and systems to communicate with each other seamlessly. From social media platforms to e-commerce websites, APIs are what make it possible for different technologies to work together harmoniously.
We’ll explore the key principles behind building scalable APIs in Laravel. We’ll dive into best practices for designing efficient interfaces that can grow alongside your application’s needs. Additionally, we’ll provide valuable tips on optimizing and securing your Laravel API so that it performs flawlessly while keeping sensitive data safe from prying eyes.
Understanding APIs
APIs, or Application Programming Interfaces, have become an integral part of modern software development. But what exactly are APIs? In simple terms, they serve as the glue that enables different applications and systems to interact with each other seamlessly. Think of APIs as a set of rules and protocols that allow developers to access certain features or functionalities of a software system.
At its core, an API acts as a mediator between two distinct pieces of software. It defines how one application can request data or perform actions from another application. This abstraction layer allows developers to build on existing functionality without having to reinvent the wheel every time.
One key advantage of using APIs is their ability to facilitate integration between different technologies. For example, social media platforms use APIs to allow third-party applications to post updates or retrieve user information securely. Similarly, e-commerce websites leverage APIs for payment gateways and shipping providers.
Understanding how APIs work is crucial for any developer looking to create scalable solutions that can communicate effectively with other systems. By mastering the art of API design and implementation, you’ll be able to unlock endless possibilities when it comes to building interconnected applications in today’s digital landscape.
Why Laravel is a popular framework for building APIs
Laravel has emerged as one of the most popular PHP frameworks for building powerful and scalable APIs. But what makes Laravel stand out among its competitors? Let’s explore some key reasons why developers prefer Laravel when it comes to API development.
Laravel provides a clean and elegant syntax, making it easy to read and write code. This not only improves developer productivity but also enhances the maintainability of the API codebase. Laravel follows MVC architectural pattern, which promotes separation of concerns and allows for better organization of code.
Another notable feature is Laravel’s robust routing system. With its expressive routing syntax, developers can easily define RESTful routes, handling HTTP verbs like GET, POST, PUT, PATCH, and DELETE effortlessly. This simplifies the process of mapping requests to specific API endpoints.
Laravel offers a rich set of built-in tools and libraries that streamline common tasks in API development. For example, Eloquent ORM simplifies database operations by providing an intuitive interface for interacting with databases. The inclusion of features like caching mechanisms and task scheduling further boosts performance and efficiency.
Laravel incorporates comprehensive testing capabilities through PHPUnit framework integration. This enables developers to write test cases for their APIs easily and ensure reliable functionality.
Lastly but importantly is the vibrant community surrounding Laravel. With active forums, online tutorials,and documentation readily available,the community support ensures quick resolutionof issuesand access to new features or enhancements made by fellow developers.
Key Principles of Scalable APIs in Laravel
It is crucial to design your API with a clear and consistent structure. This means organizing your endpoints, routes, and controllers in a logical manner that makes sense for your application. By following standard naming conventions and adhering to RESTful principles, you can ensure that your API is easy to understand and navigate.
Another important principle is separation of concerns. In Laravel, you can achieve this by leveraging the power of middleware. Middleware allows you to intercept requests and perform actions such as authentication or data validation before they reach your controller. By separating these concerns into middleware layers, you can keep your codebase clean and maintainable.
Scalability also requires careful consideration of performance optimizations. Caching frequently accessed data using tools like Redis or Memcached can greatly improve response times for your API calls. Additionally, making use of eager loading techniques like lazy loading or eager loading relationships can help minimize database queries and reduce load on your server.
Error handling is another crucial aspect of building scalable APIs. Implementing robust error handling mechanisms ensures that any unexpected issues are handled gracefully without impacting the overall performance of your API. Whether it’s returning informative error messages or logging errors for debugging purposes, taking care of these details will go a long way in providing a smooth experience for developers consuming your API.
Documentation plays an essential role in building scalable APIs with Laravel. Providing comprehensive documentation not only helps developers understand how to interact with different endpoints but also facilitates future scalability by enabling new team members or external stakeholders to quickly onboard onto the project.
By keeping these key principles in mind while building scalable APIs in Laravel, you’ll be able to create efficient and reliable interfaces that can handle increasing amounts of traffic without compromising performance or user experience.
Best Practices for Designing Scalable APIs in Laravel
When it comes to designing scalable APIs in Laravel, there are several best practices that can help ensure a smooth and efficient development process.
It’s important to carefully plan and structure your API endpoints. Keep them logical and intuitive, following RESTful principles whenever possible. This will make it easier for developers to understand and use your API effectively.
Consider implementing pagination for large data sets. By breaking up the response into smaller chunks, you can improve performance and prevent potential timeouts or memory issues.
Another key practice is versioning your APIs. As your application evolves over time, it’s crucial to maintain backward compatibility with existing clients while introducing new features or making changes. Versioning allows you to gracefully transition between different versions of the API without disrupting users.
Proper error handling is essential for any robust API design. Implement meaningful error messages with appropriate HTTP status codes so that developers using your API can easily troubleshoot issues when they arise.
Authentication and authorization should be given careful consideration. Use Laravel’s built-in authentication mechanisms such as JWT or OAuth2 to secure access to your APIs effectively.
Always include comprehensive documentation for your APIs. This should cover everything from endpoint descriptions and request/response formats to authentication requirements and rate limiting policies. Good documentation reduces confusion among developers who consume your API and encourages adoption.
By adhering closely to these best practices throughout the design process of a scalable Laravel API project, you’ll set yourself up for success by creating an efficient yet user-friendly system that meets the needs of both developers and end-users alike!
Tips for Optimizing and Securing Your Laravel API
When building a Laravel API, optimizing and securing it should be at the top of your priority list. This ensures that your API performs efficiently and protects sensitive data from unauthorized access or attacks.
One important tip for optimizing your Laravel API is to utilize caching. By implementing caching mechanisms such as Redis or Memcached, you can store frequently accessed data in memory, reducing the load on your database and improving response times.
Another optimization technique is to implement pagination when returning large sets of data. Instead of retrieving all records at once, paginate the results to limit the amount of data sent over the network and improve performance.
To enhance security in your Laravel API, always validate user input to prevent potential vulnerabilities like SQL injection or cross-site scripting (XSS) attacks. Utilize Laravel’s built-in validation features or custom validation rules to ensure that only valid and safe input is accepted.
Consider implementing rate limiting to protect against abuse or excessive requests. Set limits on how many requests a client can make within a certain time frame to prevent denial-of-service (DoS) attacks and maintain optimal performance for other users.
Apply authentication and authorization mechanisms to restrict access based on user roles and permissions. Laravel provides robust tools like Passport for OAuth2 authentication or JWT tokens for stateless authentication.
Keep your dependencies up-to-date by regularly updating packages used in your API. New releases often include bug fixes, security patches, and performance improvements that can benefit your application.
Harness the Power of Laravel to Build Scalable and Efficient APIs
Laravel, with its robust features and elegant syntax, provides developers with a powerful tool for building scalable and efficient APIs. By following key principles and best practices, you can create APIs that are not only capable of handling heavy loads but also maintainable and secure.
One of the key advantages of using Laravel for API development is its modularity. With Laravel’s modular structure, you can easily separate different components of your API into smaller modules or packages. This allows for better organization, code reusability, and easier maintenance in the long run.
Laravel’s built-in support for caching mechanisms like Redis or Memcached enables developers to optimize their API performance by storing frequently accessed data in memory. This drastically reduces response times and improves overall scalability.
Another important aspect when building scalable APIs is validation. Laravel offers a comprehensive validation system that allows you to validate incoming requests effortlessly. By properly validating user input before processing it further, you can prevent potential security vulnerabilities or errors from occurring.
Laravel’s ORM tool called Eloquent simplifies database operations by providing an intuitive way to interact with databases using object-oriented syntax. With Eloquent’s query builder methods like eager loading or lazy loading relationships, you can efficiently retrieve data without making unnecessary queries.
When it comes to securing your API endpoints, Laravel offers various mechanisms such as JWT authentication or OAuth2 integration out-of-the-box. These authentication methods help ensure that only authorized users can access sensitive resources within your API.
To optimize the performance of your Laravel API even further, consider implementing techniques like request throttling or rate limiting to prevent abuse or excessive usage by individual clients. Utilizing caching strategies at different levels helps reduce response times significantly.