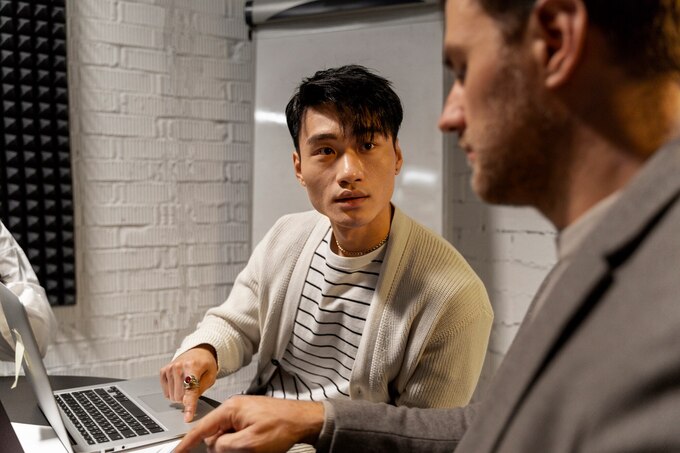
Laravel has become a favorite among developers for its elegant syntax and powerful features. At the heart of Laravel’s capabilities lies Eloquent, an ORM (Object-Relational Mapping) tool that simplifies database interactions. But as your application grows, so do the complexities of managing relationships between models.
Navigating these relationships efficiently is crucial. Not only does it enhance performance, but it also leads to better user experiences. Optimizing database queries can make a notable difference in load times and responsiveness—elements that are essential in today’s fast-paced web environment.
Understanding the Importance of Optimizing Database Queries
Optimizing database queries is crucial for any application. Slow queries can lead to poor user experiences and increased load times. This can frustrate users, driving them away.
When you optimize your queries, you not only improve speed but also reduce server strain. A well-optimized query uses fewer resources, allowing your application to handle more traffic effectively.
Efficient queries contribute to faster data retrieval. When every millisecond counts, the difference between a slow and fast query can significantly impact overall performance.
Understanding how your database interacts with Laravel’s Eloquent ORM helps streamline these processes further. You gain insights into what works best for your specific use case.
A focus on optimization ensures that you’re building scalable applications ready for growth. As projects expand, so do the demands placed on databases—properly optimized queries help manage this evolving landscape seamlessly.
Techniques for Speeding Up Eloquent Queries
Optimizing Eloquent queries is crucial for enhancing application performance. Start by using the `select` method to retrieve only the necessary columns instead of fetching entire models. This reduces memory usage and speeds up data retrieval.
Consider eager loading with `with()` to minimize the N+1 query problem. By preloading relationships, you can fetch related records in fewer database calls, which significantly improves load times.
Utilize caching strategies as well. Storing frequently accessed data can dramatically decrease response times and lessen database load.
Make use of indexing on your database tables where applicable. Properly indexed columns enhance search efficiency, making lookups faster than ever.
Explore chunking results with methods like `chunk()` or `cursor()`. These methods allow processing large datasets without overwhelming server resources or causing timeouts.
Advanced Eloquent Relationships: HasManyThrough and MorphTo
Laravel’s Eloquent ORM offers powerful tools for managing complex relationships. Two advanced relationship types, HasManyThrough and MorphTo, stand out for their flexibility.
HasManyThrough allows you to access distant relationships in a streamlined way. For instance, if you have users who can write posts that are tagged with categories, this setup simplifies fetching all categories associated with a user’s posts. Instead of writing multiple queries or joining tables manually, Eloquent handles it beautifully under the hood.
On the other hand, MorphTo provides polymorphic relations that let models belong to more than one type of model on a single association. Imagine a comment system where comments can belong either to posts or videos. With MorphTo, you can fetch these comments uniformly without convoluted logic in your codebase.
These features not only make data retrieval efficient but also enhance code readability and maintainability across your Laravel projects.
Case Studies: Real-Life Examples of Improved Performance Using Advanced Eloquent Relationships
A popular e-commerce platform faced sluggish loading times due to complex Eloquent queries. The developers implemented the `hasManyThrough` relationship to optimize data retrieval for orders and products. This change reduced database calls, significantly improving page load speeds.
In another instance, a social media application struggled with user notifications. By leveraging the `morphTo` relationship, they streamlined how different notification types were fetched. Instead of multiple queries for each type, a single query retrieved all relevant notifications efficiently.
These real-life examples illustrate how advanced relationships can transform performance metrics in Laravel applications. Developers noted quicker response times and enhanced user experiences after implementing these techniques. Embracing such strategies has become essential for modern web applications aiming to scale effectively without sacrificing speed or functionality.
The Benefits of Utilizing Advanced Eloquent Relationships in Laravel Projects
Leveraging advanced Eloquent relationships can significantly enhance the performance of your Laravel applications. By using techniques such as `HasManyThrough` and `MorphTo`, you streamline database queries, reduce overhead, and improve load times.
These optimizations not only make your code cleaner but also lead to a better user experience. Faster response times keep users happy and engaged. Moreover, efficient database interactions allow developers to focus on building features rather than troubleshooting slow queries.
As you integrate these advanced relationships into your projects, consider real-life case studies that highlight tangible improvements in speed and efficiency. Adopting these practices will set your Laravel applications apart in terms of performance and responsiveness. Embracing advanced Eloquent relationships is a step toward achieving scalable and maintainable code for future growth.