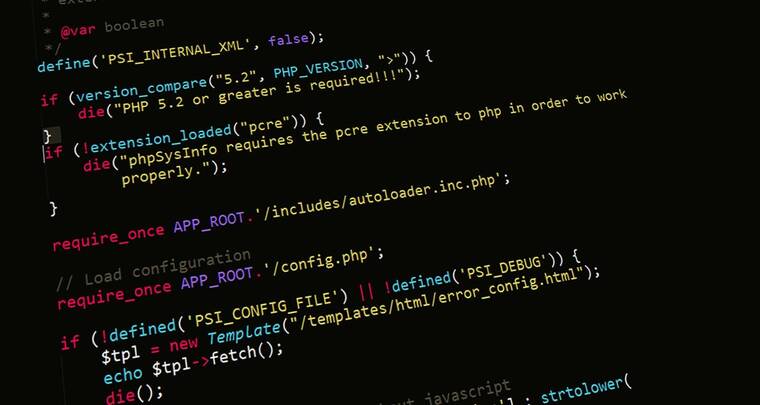
Welcome to the world of Laravel, where elegance and efficiency meet in perfect harmony! With its intuitive syntax, robust features, and a vibrant community backing it up, Laravel has skyrocketed in popularity over the years. But with great power comes great responsibility – especially when it comes to security.
Security is of paramount importance for any web application. From protecting user data to preventing unauthorized access, ensuring the safety and integrity of your Laravel application should be at the top of your priority list. We will explore some best practices and techniques to scale your Laravel application securely.
Importance of Security in Web Development
Web development plays a crucial role in the success of businesses and organizations. From e-commerce websites to online banking platforms, the internet has become an integral part of our daily lives. However, with increased connectivity comes greater risks and vulnerabilities.
Security is paramount when it comes to web development. Without adequate measures in place, sensitive user data can be compromised, leading to severe consequences such as identity theft or financial loss. This is where secure coding practices come into play.
By implementing strong security measures during the development process, developers can ensure that their applications are protected from potential attacks. This includes safeguarding against common threats like cross-site scripting (XSS), SQL injection, and session hijacking.
Authentication and authorization mechanisms should be implemented to verify user identities and control access to sensitive information. By incorporating robust password policies and multi-factor authentication techniques, developers can significantly reduce the risk of unauthorized access.
Securing database interactions is another essential aspect of web application security. Proper input validation must be performed on user-submitted data before it is stored or processed by the application to prevent malicious code execution or unauthorized database queries.
To promote secure coding practices in Laravel applications specifically, developers should follow best practices recommended by Laravel itself. These include utilizing built-in features like CSRF protection tokens for form submissions and enforcing HTTPS connections for enhanced encryption.
Regular updates and patches should be applied promptly to address any known security vulnerabilities within Laravel or its dependencies. Keeping up with these updates ensures that your application stays protected against emerging threats.
Common Security Risks in Laravel Applications
When it comes to developing web applications, security should always be a top priority. Laravel, being one of the most popular PHP frameworks, is renowned for its robustness and security features. However, like any other application, Laravel is not immune to common security risks.
One of the most prevalent risks in Laravel applications is cross-site scripting (XSS). This occurs when an attacker injects malicious scripts into a website that are then executed by unsuspecting users. To prevent XSS attacks, developers must ensure that user input is properly validated and sanitized before displaying it on the website.
Another common risk is SQL injection. This occurs when an attacker manipulates database queries through user-supplied data. To mitigate this risk, developers should use Laravel’s built-in query builder or ORM instead of writing raw SQL queries.
Insecure direct object references can also pose a threat to Laravel applications. This happens when developers expose internal implementation details such as database IDs in URLs or forms. Attackers can exploit this vulnerability by manipulating these references to access unauthorized resources.
Inadequate authentication and authorization mechanisms can leave your application vulnerable to attacks. It’s crucial to implement strong password policies, enforce secure session management practices, and restrict access based on roles and permissions.
Insecure file uploads can open doors for attackers to upload malicious files onto your server. Developers need to validate file types and sizes while ensuring that uploaded files are stored outside the public directory or restricted from execution.
Implementing Authentication and Authorization
Implementing Authentication and Authorization is crucial for ensuring the security of Laravel applications. By enforcing proper user authentication, you can prevent unauthorized access to sensitive data and protect your users’ information.
In Laravel, authentication is made simple with built-in features like the Auth facade and pre-built controllers. You can easily implement login and registration functionality by leveraging these tools. Additionally, Laravel provides middleware that allows you to restrict access to certain routes based on user roles or permissions.
To further enhance security, it’s important to properly store passwords using hashing algorithms. Laravel offers bcrypt as the default password hash algorithm, which ensures that passwords are securely encrypted before being stored in the database.
Authorization plays a key role in controlling what actions users can perform within an application. By defining authorization policies and using gates or policies provided by Laravel, you can easily manage permissions at various levels of granularity.
By implementing strong authentication mechanisms and carefully managing authorization levels, you can significantly reduce the risk of unauthorized access or data breaches in your Laravel applications.
Securing Database Interactions
Securing Database Interactions is a critical aspect of building secure Laravel applications. When it comes to dealing with databases, there are several best practices that developers should follow to ensure the protection of sensitive data.
One fundamental principle is to always use parameterized queries or prepared statements instead of directly embedding user input into SQL queries. This helps prevent SQL injection attacks by separating the SQL code from the data being passed in.
Another essential practice is to validate and sanitize user inputs before interacting with the database. Laravel provides convenient validation methods that can be used to validate and sanitize input data, helping to prevent malicious code or unexpected inputs from causing harm.
It’s crucial to properly handle errors and exceptions related to database interactions. Displaying detailed error messages can inadvertently expose sensitive information about your application’s structure or database schema. Instead, log these errors securely without revealing any confidential details.
By following these best practices for securing database interactions in Laravel applications, developers can significantly reduce the risk of unauthorized access, data corruption, or leakage through vulnerabilities originating at this crucial layer.
Best Practices for Secure Coding
When it comes to secure coding practices in Laravel, there are several key measures that developers should follow. These best practices not only help protect the application from potential vulnerabilities but also ensure that user data remains safe and confidential.
Keeping your Laravel framework up to date is crucial for security. Regularly updating to the latest version ensures that any security patches or fixes are applied, reducing the risk of known vulnerabilities being exploited.
Another important practice is validating all user input thoroughly. This includes implementing server-side validation for form submissions and using Laravel’s built-in validation features. By validating user input, you can prevent common attacks such as SQL injection or cross-site scripting (XSS).
It’s essential to use strong encryption techniques when storing sensitive data in databases. Laravel provides convenient methods for encrypting passwords and other sensitive information using bcrypt hashing algorithm.
Securing API endpoints is another critical aspect of secure coding in Laravel applications. Implementing authentication mechanisms such as JWT tokens or OAuth2 can help verify the identity of users accessing APIs and restrict unauthorized access.
By following these best practices for secure coding in Laravel applications, developers can significantly reduce the risk of common security vulnerabilities while ensuring a safer experience for their users.
Additional Security Measures for Laravel Applications
In addition to implementing authentication and authorization, there are several other security measures that can be taken to ensure the robustness of Laravel applications. These additional measures further enhance the overall security posture and protect against various types of attacks.
One important aspect is input validation, which helps prevent malicious code or unexpected data from being processed by the application. Laravel provides built-in validation rules that can be easily applied to user inputs, ensuring they meet specific criteria before being accepted.
Another crucial measure is implementing secure session management. This involves encrypting session data and using secure cookies with proper expiration times. By doing so, it becomes more challenging for attackers to hijack sessions and gain unauthorized access.
Securing sensitive information such as passwords or API keys is also vital in maintaining a secure Laravel application. It is recommended to store passwords securely by using strong hashing algorithms like bcrypt. Additionally, sensitive configuration files should be protected from unauthorized access by setting appropriate file permissions.
Continuous monitoring of logs and system activities can provide early detection of any suspicious behavior or potential security issues. This allows administrators to promptly respond and take necessary actions before any significant damage occurs.
By incorporating these additional security measures into Laravel applications, developers can significantly reduce the risk of successful attacks while providing users with a safe browsing experience.
Conclusion
In today’s digital age, security is of utmost importance when it comes to web development. With the increasing number of cyber threats and attacks, developers need to be vigilant in protecting their applications from potential vulnerabilities. Laravel, with its robust framework and comprehensive security features, has emerged as a popular choice among developers.
Throughout this article, we have discussed some of the common security risks that can affect Laravel applications and explored best practices for mitigating these risks. We emphasized the importance of implementing authentication and authorization mechanisms to ensure only authorized users have access to sensitive data.
Securing database interactions is crucial in preventing unauthorized access or manipulation of data. By following secure coding practices such as input validation, output escaping, proper error handling, and using parameterized queries or prepared statements when interacting with databases, developers can significantly reduce the risk of SQL injection attacks.
We highlighted other essential security measures like enabling HTTPS for secure communication between clients and servers and regularly updating dependencies to patch any known vulnerabilities.
By prioritizing security throughout the entire development lifecycle of your Laravel applications using these recommended practices outlined here you’ll be well-equipped to scale securely while providing a safe browsing experience for your users.