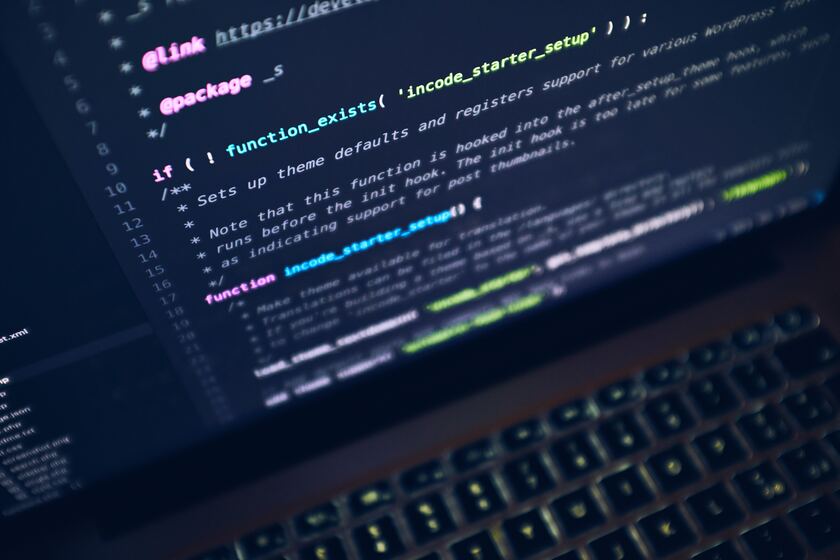
Welcome to the exciting world of Laravel, where building robust web applications is a breeze! Whether you’re a seasoned developer or just starting out on your coding journey, you’ll quickly discover that Laravel offers an elegant and powerful framework for creating scalable applications.
But what if I told you there’s a secret weapon that can take your Laravel projects to new heights of scalability? Enter Redis, the lightning-fast in-memory data structure store. In this blog post, we’ll explore how Redis can supercharge your Laravel applications and help them handle massive amounts of traffic without breaking a sweat.
Understanding Redis and its Benefits for Laravel Applications
Redis is a powerful and versatile in-memory data structure store that can bring significant benefits to Laravel applications. It acts as a cache and provides high-speed data access, making it an ideal choice for improving the performance and scalability of your Laravel projects.
One of the key advantages of using Redis with Laravel is its ability to reduce database load. By caching frequently accessed data in memory, Redis allows you to retrieve information quickly without having to make repeated database queries. This not only improves response times but also reduces the strain on your database server, ultimately enhancing the overall scalability and efficiency of your application.
Another benefit of Redis is its support for pub/sub messaging patterns. With Redis pub/sub functionality integrated into your Laravel application, you can easily implement real-time features such as chat systems or live notifications. This enables seamless communication between different components of your application, enhancing user experience and engagement.
Redis offers advanced features like sorted sets and geospatial indexes that enable efficient querying and sorting operations on large datasets. These capabilities can be particularly useful when working with complex data structures or performing calculations based on proximity or ranking.
By leveraging these benefits offered by Redis, you can create highly scalable Laravel applications that deliver exceptional performance even under heavy loads. In the next section, we will explore how to implement Redis in a step-by-step guide for your Laravel project. Stay tuned!
How Redis Enhances Scalability in Laravel Applications
Redis is a powerful tool that can greatly enhance the scalability of Laravel applications. By utilizing Redis as a caching layer, developers can significantly improve the performance and responsiveness of their applications.
One key benefit of using Redis in Laravel applications is its ability to store and retrieve data in-memory. This means that data can be accessed much faster than if it were stored on disk. As a result, response times are reduced, allowing for more concurrent requests to be handled by the application.
Redis offers various data structures and features that can further optimize scalability. For example, the use of sorted sets allows for efficient retrieval of data based on ranking or scoring criteria. This can be particularly useful in scenarios where real-time leaderboards or rankings need to be updated frequently.
Redis supports pub/sub messaging which enables asynchronous communication between different components of an application. This decoupling of functionality allows for greater flexibility and scalability as individual components can scale independently without affecting others.
Redis provides several mechanisms for improving scalability in Laravel applications. From caching frequently accessed data to facilitating asynchronous communication between components, Redis plays a crucial role in building scalable and high-performance Laravel applications.
Step-by-Step Guide to Implementing Redis in a Laravel Application
Implementing Redis in a Laravel application can greatly enhance its performance and scalability. Here is a step-by-step guide to help you get started.
First, make sure that Redis is installed on your server or local machine. You can either download and install it manually or use a package manager like Homebrew for macOS or apt-get for Ubuntu.
Next, add the Redis service provider to your Laravel configuration file. Open the config/app.php file and locate the ‘providers’ array. Add the following line:
RedisServiceProvider::class
Now, you need to configure the connection settings for Redis. Open the config/database.php file and find the ‘redis’ array. Update the ‘default’ value to specify which Redis connection should be used by default.
To start using Redis in your application code, you will first need to create an instance of the Redis class. You can do this by calling `app(‘redis’)` or using Dependency Injection.
Once you have an instance of the Redis class, you can start using its methods to interact with key-value data stored in Redis.
If you want to store some data in a cache using redis, simply call `$redis->set(‘key’, ‘value’);`.
If you need more advanced features like caching query results or session management with redis, there are additional packages available that integrate seamlessly with Laravel.
Remember to always handle exceptions when working with redis operations as failures may occur due network issues or other reasons beyond your control.
Best Practices for Using Redis in a Laravel Application
When it comes to using Redis in your Laravel application, there are certain best practices that can help you make the most out of this powerful tool. Here are some key tips to keep in mind:
1. Use caching wisely: Redis is excellent for caching frequently accessed data and reducing database queries. However, it’s important not to overuse caching as it can lead to stale data. Identify the parts of your application that would benefit the most from caching and implement it strategically.
2. Utilize pub/sub feature: Redis provides a publish/subscribe mechanism which allows you to broadcast messages between different components of your application. This can be particularly useful when building real-time features such as chat systems or notification systems.
3. Optimize memory usage: Redis stores all data in-memory, so it’s crucial to monitor and optimize memory usage. Set appropriate expiration times for cached items and remove unused keys regularly to prevent memory overload.
4. Avoid unnecessary round trips: When interacting with Redis, try to minimize the number of round trips by utilizing pipelining or Lua scripting where possible. This helps reduce network latency and improves overall performance.
5. Secure your Redis instance: Protecting your Redis instance is essential to prevent unauthorized access or potential security breaches. Implement authentication mechanisms and restrict external access if necessary.
6. Monitor performance: Regularly monitor the performance of your Redis server using tools like redis-cli or third-party monitoring solutions. Keep an eye on metrics such as memory usage, CPU utilization, and response times to ensure optimal performance.
Incorporating these best practices will help you harness the full potential of Redis within your Laravel application while ensuring scalability, reliability, and security.
Real-Life Examples of Successful Scalable Laravel Applications with Redis
1. E-commerce Platforms: Many e-commerce platforms have leveraged the power of Laravel and Redis to handle high volumes of traffic and ensure smooth user experiences. By caching frequently accessed product data, session information, and even search results using Redis, these platforms can scale their applications seamlessly.
2. Social Media Networks: Popular social media networks like Instagram have utilized Laravel in combination with Redis to handle millions of active users without compromising performance. With Redis’s ability to store session data efficiently and handle real-time notifications, these platforms can deliver timely updates and maintain a seamless user experience.
3. On-demand Service Apps: On-demand service apps such as Uber or TaskRabbit require real-time tracking and efficient handling of requests. By integrating Laravel with Redis for caching driver locations, ride histories, or task details, these apps can provide quick responses and manage large-scale operations smoothly.
4. Gaming Platforms: Online gaming platforms often face challenges when it comes to scalability due to the fast-paced nature of gameplay. By utilizing Laravel’s robust framework along with Redis for storing game state information or leaderboard rankings, developers can build scalable gaming applications that offer uninterrupted gameplay experiences.
5. Content Management Systems (CMS): CMS solutions built on top of Laravel benefit greatly from incorporating Redis into their architecture. By caching frequently accessed content, database queries are minimized, resulting in faster load times for websites or web applications that rely on dynamic content management systems.
Why Every Laravel Developer Should Consider Using Redis for Scalability
Redis is undoubtedly a powerful tool that can significantly enhance the scalability of Laravel applications. Its ability to store and retrieve data quickly, its support for various data structures, and its seamless integration with Laravel make it an ideal choice for developers looking to build scalable applications.
By implementing Redis in a Laravel application, developers can offload time-consuming tasks such as caching, session management, and job queues to Redis. This not only improves overall performance but also allows the application to handle more concurrent requests without any hiccups.
Redis offers features like pub/sub messaging and real-time analytics that can further enhance the functionality of a Laravel application. With pub/sub messaging, developers can easily implement real-time notifications or chat functionalities in their applications. Real-time analytics allow developers to gather valuable insights about their application’s performance and user behavior.
In addition to these benefits, using Redis in a Laravel application follows best practices for building scalable software. It promotes separation of concerns by decoupling caching logic from business logic and enables horizontal scaling by allowing multiple instances of the application to share a common cache store.
Every Laravel developer should seriously consider incorporating Redis into their projects if they want to build highly scalable applications that can handle large amounts of traffic efficiently. The ease of use, flexibility, and numerous benefits offered by Redis make it an invaluable tool in the arsenal of any developer working with Laravel framework.