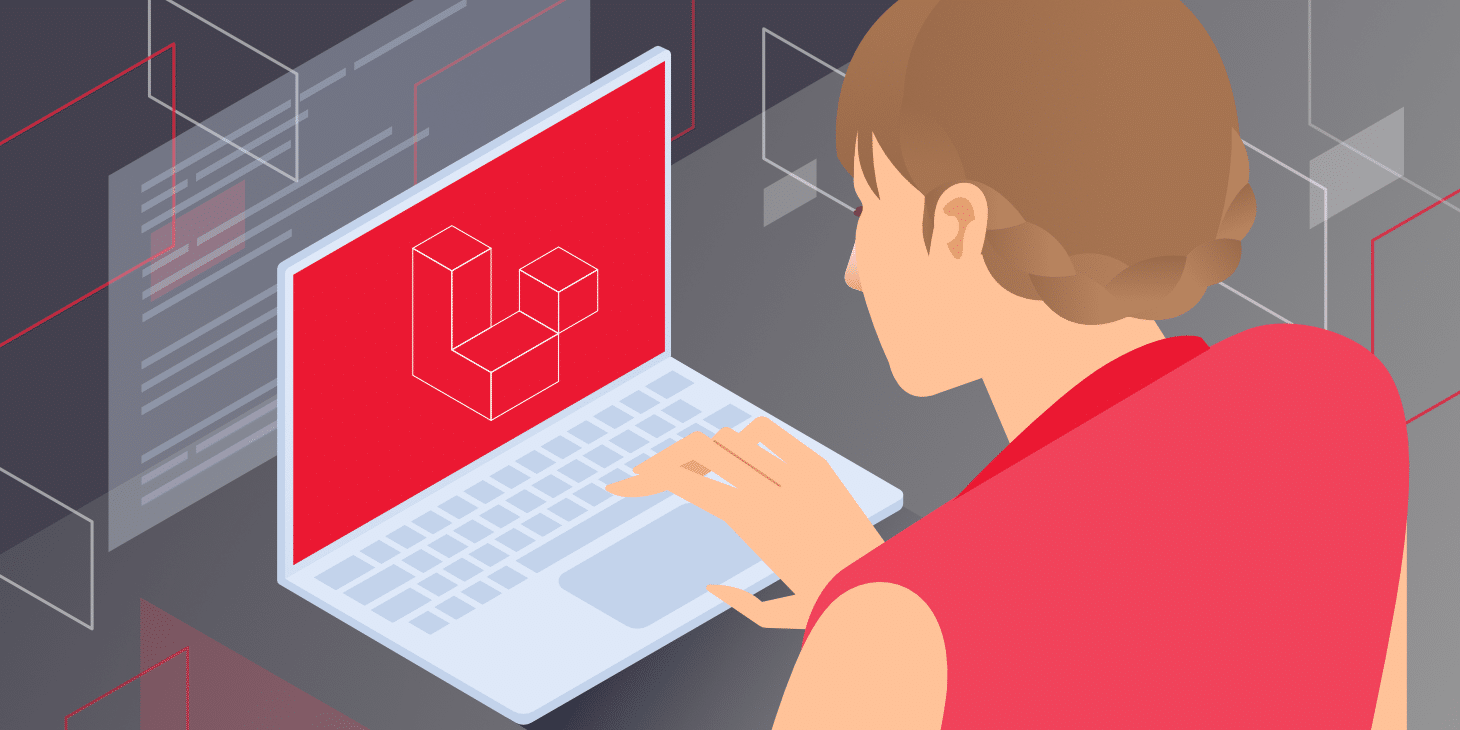
Are you ready to take your Laravel application to the next level? Scalability is a crucial aspect of web development that can make or break your success in today’s fast-paced digital landscape. With thousands of users accessing your website simultaneously, it’s essential to ensure that it can handle the load without compromising performance. That’s where Laravel comes in.
Laravel, one of the most popular PHP frameworks, offers a robust set of scalability features that allow you to build applications capable of handling high traffic volumes effortlessly. In this blog post, we will explore the best practices for scaling a Laravel application and maximizing its potential.
So grab your coffee and get ready to dive into the world of scalability with Laravel! Whether you’re a seasoned developer or just starting out, these tips and techniques will help you master scalability like never before. Let’s get started!
Understanding Scalability in Web Development
Understanding scalability is crucial in web development as it determines how well your application can handle increasing amounts of traffic and user interactions. In simple terms, scalability refers to the ability of a system to handle growth without sacrificing performance or reliability.
When it comes to web applications, scalability is often measured in terms of handling concurrent users, processing speed, database queries, and resource utilization. As more users access your website simultaneously, the demand for resources increases. If your application cannot scale effectively, it may result in slow response times or even crashes under heavy loads.
Scalability can be achieved through various techniques such as caching data, optimizing database queries, using asynchronous processing with queues, and employing horizontal or vertical scaling methods. These practices ensure that your application can efficiently distribute workload across multiple servers or increase its capacity by upgrading hardware resources.
It’s important to note that scalability should not be an afterthought but rather considered from the early stages of development. By designing a scalable architecture and following best practices throughout the development process, you can future-proof your application and ensure its smooth operation as user demands grow.
Understanding scalability is essential for building successful web applications capable of handling increasing traffic volumes without compromising performance. By implementing proper strategies and utilizing tools like Laravel’s built-in features for scaling applications efficiently, developers can create robust systems that adapt seamlessly to changing user needs. So make sure you prioritize scalability when developing your next project!
Introducing Laravel and its Scalability Features
Laravel, a popular PHP framework, has gained immense popularity among developers due to its robust features and ease of use. One of the standout features of Laravel is its ability to handle scalability with grace. As your application grows in size and complexity, Laravel provides several tools and techniques to ensure that it can handle increasing traffic without compromising performance.
One notable feature in Laravel is its support for caching. By utilizing various caching mechanisms such as file-based or database-driven caching, you can significantly improve the response time of your application. Caching frequently accessed data or rendered views can reduce the load on your server and enhance overall performance.
Another key aspect for scaling Laravel applications efficiently is by leveraging queues. Queues allow you to offload time-consuming tasks from the main execution flow, enabling asynchronous processing. By using a message broker like Redis or RabbitMQ, you can handle heavy workloads more efficiently while keeping your application responsive.
When it comes to horizontal scaling, Laravel offers seamless integration with cloud services like AWS or Google Cloud Platform. With just a few configuration changes, you can distribute your application across multiple servers easily. This allows for increased capacity handling as well as better fault tolerance.
On the other hand, vertical scaling involves upgrading hardware resources such as CPU power or memory on a single server instance running Laravel. By optimizing code efficiency and utilizing resource-intensive components sparingly when necessary, vertical scaling becomes an effective approach for managing peak loads without major architectural changes.
To ensure smooth scalability in production environments, monitoring plays a crucial role in detecting potential bottlenecks or issues early on before they impact user experience negatively. Tools like New Relic or Datadog provide insights into system metrics allowing proactive optimization measures based on real-time data analysis.
Best Practices for Scaling a Laravel Application
When it comes to scaling a Laravel application, there are several best practices that can help ensure your application performs optimally as user traffic increases. Here are some key tips to consider:
1. Use Caching: Implementing caching techniques can dramatically improve the performance of your Laravel application. By storing frequently accessed data in cache rather than querying the database each time, you can reduce response times and alleviate server load.
2. Optimize Database Queries: Efficiently written database queries are essential for scalability. Make use of indexing, eager loading, and query optimization techniques such as selecting only necessary columns to minimize overhead and improve response times.
3. Utilize Queues: Laravel’s built-in queue system allows you to offload time-consuming tasks to background workers, keeping your application responsive even during heavy loads. By decoupling these tasks from the main request cycle, you can enhance scalability without impacting user experience.
4. Scale Horizontally and Vertically: Horizontal scaling involves adding more servers or instances to distribute workload across multiple machines, while vertical scaling focuses on increasing resources within a single server or instance. Consider which approach best suits your application’s needs.
5. Monitor Performance Metrics: Regularly monitor various performance metrics like response times, CPU usage, memory consumption, etc., using tools like New Relic or Datadog. This helps identify bottlenecks and areas for improvement before they impact users.
By following these best practices and continually optimizing your Laravel application’s infrastructure and codebase as needed, you’ll be well-equipped to handle increased traffic effectively without sacrificing performance or stability!
Caching Techniques to Improve Performance
One of the key strategies for improving performance in a Laravel application is through effective caching techniques. Caching involves storing frequently accessed data or computations in memory, allowing subsequent requests to be served faster.
Laravel provides robust caching mechanisms out of the box, making it easy to implement. The most common type of cache used in Laravel is the built-in file-based cache driver. This driver stores cached data as serialized PHP files on disk.
Laravel also supports other popular caching methods such as Redis and Memcached. These can significantly enhance performance by storing cached data in memory, reducing database queries and computation time.
To take advantage of caching in your Laravel application, you can use various methods provided by the framework’s Cache facade. For instance, you can store a value in the cache using the `put` method and retrieve it later using the `get` method.
Another powerful feature offered by Laravel is cache tagging. With this technique, you can assign tags to cached items and easily invalidate or clear all items associated with a particular tag when needed. This allows for more granular control over cached data and helps keep it up-to-date.
By strategically implementing caching techniques throughout your application, you can greatly improve its overall performance and scalability. However, it’s important to strike a balance between excessive caching and not enough caching.
Remember that different parts of your application may benefit from different types of caches or even custom cache implementations tailored specifically for their needs. It’s essential to analyze your specific use cases carefully and choose an appropriate approach accordingly.
Using Queues for Efficient Processing
One of the key challenges in scaling a Laravel application is handling large volumes of requests and ensuring that they are processed efficiently. This is where queues come into play.
Queues allow you to offload time-consuming tasks from your main application flow, creating a separate queue for processing them asynchronously. By doing so, you can ensure that your application remains responsive even when there are heavy workloads or long-running processes.
Laravel provides an intuitive and powerful queuing system that makes it easy to implement this functionality. The framework supports multiple queue drivers, including database, Redis, Amazon SQS, and Beanstalkd.
To use queues effectively, start by identifying the tasks that can be processed asynchronously. These could include sending emails, generating reports, or performing complex calculations. By placing these tasks into a job queue instead of executing them immediately within the request cycle, you can significantly improve response times and overall performance.
When using queues with Laravel, it’s important to design your jobs carefully. Each job should encapsulate a specific task or action and be designed to run independently of other jobs in the queue. This allows for better scalability as individual jobs can be processed on different servers or instances simultaneously.
Consider prioritizing your queued jobs based on their importance or urgency. Laravel allows you to assign priorities to each job so that critical tasks are processed before lower-priority ones.
Monitoring and managing your queues is also crucial for efficient processing. Laravel provides tools such as Horizon which offer real-time monitoring of your queues along with various configuration options for optimizing their performance.
Horizontal and Vertical Scaling with Laravel
Horizontal and vertical scaling are two common approaches to handle the increasing demands of a Laravel application. Let’s dive into each method and explore how they can help improve scalability.
Horizontal scaling involves adding more servers or instances to distribute the workload across multiple machines. By doing this, you can accommodate higher traffic volumes without overloading a single server. This approach is suitable when your application needs to handle a large number of concurrent requests.
On the other hand, vertical scaling focuses on upgrading the hardware resources of an existing server. By increasing CPU power, memory capacity, or disk space, you can enhance the performance capabilities of your Laravel application. This approach is beneficial when you have bottlenecks in resource usage and need to optimize efficiency.
In Laravel, both horizontal and vertical scaling can be achieved using various tools and techniques. For horizontal scaling, you may consider utilizing load balancers to evenly distribute incoming requests among multiple servers or implementing a cluster management system like Kubernetes. Additionally, using database replication or caching mechanisms such as Redis can also aid in achieving efficient horizontal scalability.
Vertical scaling with Laravel involves optimizing your codebase for improved resource utilization by leveraging features like eager loading relationships instead of lazy loading and minimizing unnecessary database queries through query optimization techniques. Additionally, configuring web server settings appropriately will help maximize performance gains from hardware upgrades.
Remember that choosing between horizontal and vertical scaling depends on factors such as expected user traffic patterns, budget constraints, infrastructure setup complexity, and future growth projections for your application.
Implementing proper monitoring tools along with automated alerts will allow you to identify potential scalability issues proactively before they impact user experience negatively. Regularly reviewing logs generated by your application helps pinpoint any underlying performance bottlenecks that need attention.
Scalability is an ongoing process that requires continuous evaluation and adjustment based on changing requirements over time. By understanding different strategies available within Laravel framework for horizontal & vertical Scaling we gain greater control over our applications’ growth trajectory while ensuring optimal performance levels are maintained.
Monitoring and Troubleshooting for Scalability Issues
Monitoring and troubleshooting are crucial aspects of managing scalability issues in a Laravel application. Without proper monitoring, it becomes challenging to identify bottlenecks and address them effectively. Fortunately, Laravel offers several tools and techniques that can help streamline this process.
One of the key tools for monitoring a Laravel application is the built-in debug bar. This handy feature provides detailed information about the queries executed, memory usage, HTTP requests, and more. By analyzing this data, developers can pinpoint performance issues and optimize their code accordingly.
Laravel also supports integration with popular logging services like Monolog or Loggly. These services allow developers to monitor their application’s logs in real-time and set up alerts for critical errors or performance thresholds.
Another important aspect of monitoring scalability is load testing. By simulating high traffic scenarios using tools like Apache JMeter or Siege, developers can gauge how well their application handles increased loads. Load testing helps identify potential bottlenecks early on so that they can be addressed proactively.
When it comes to troubleshooting scalability issues, having access to comprehensive error tracking is essential. Services like Bugsnag or Sentry provide real-time error reporting along with detailed stack traces which assist in identifying the root cause of problems quickly.
Furthermore, profiling tools such as Blackfire enable developers to analyze their code’s performance by measuring execution time and memory consumption at different stages of request processing. Profiling helps detect areas where improvements can be made for better scalability.
Effective monitoring and troubleshooting play a vital role in ensuring the smooth scaling of a Laravel application. With the right tools and practices in place, developers can proactively address potential bottlenecks before they become major roadblocks to growth.
Conclusion
Scalability is a crucial aspect of web development, especially when it comes to handling large amounts of traffic and data. In this article, we have explored the various best practices for scaling a Laravel application.
We started by understanding the concept of scalability in web development and its importance. Then, we introduced Laravel and its built-in features that make it an excellent choice for scalable applications.
Next, we delved into the best practices for scaling a Laravel application. We discussed caching techniques to improve performance by reducing database queries and utilizing cache drivers effectively. We also explored using queues for efficient processing of time-consuming tasks.
We examined horizontal and vertical scaling with Laravel. Horizontal scaling allows us to handle increased traffic by distributing the load across multiple servers or instances, while vertical scaling involves upgrading server resources like CPU or RAM to handle more requests concurrently.
We touched upon monitoring and troubleshooting for scalability issues. It is vital to continuously monitor your application’s performance and identify any bottlenecks or potential areas for improvement.
Mastering scalability in Laravel requires a combination of best practices such as effective caching techniques, efficient task processing with queues, proper horizontal/vertical scaling strategies, and proactive monitoring/troubleshooting measures.
By implementing these techniques in your Laravel projects, you can ensure that your applications are ready to handle increasing demands without sacrificing performance or user experience. So go ahead and leverage these best practices to take your Laravel applications to new heights!