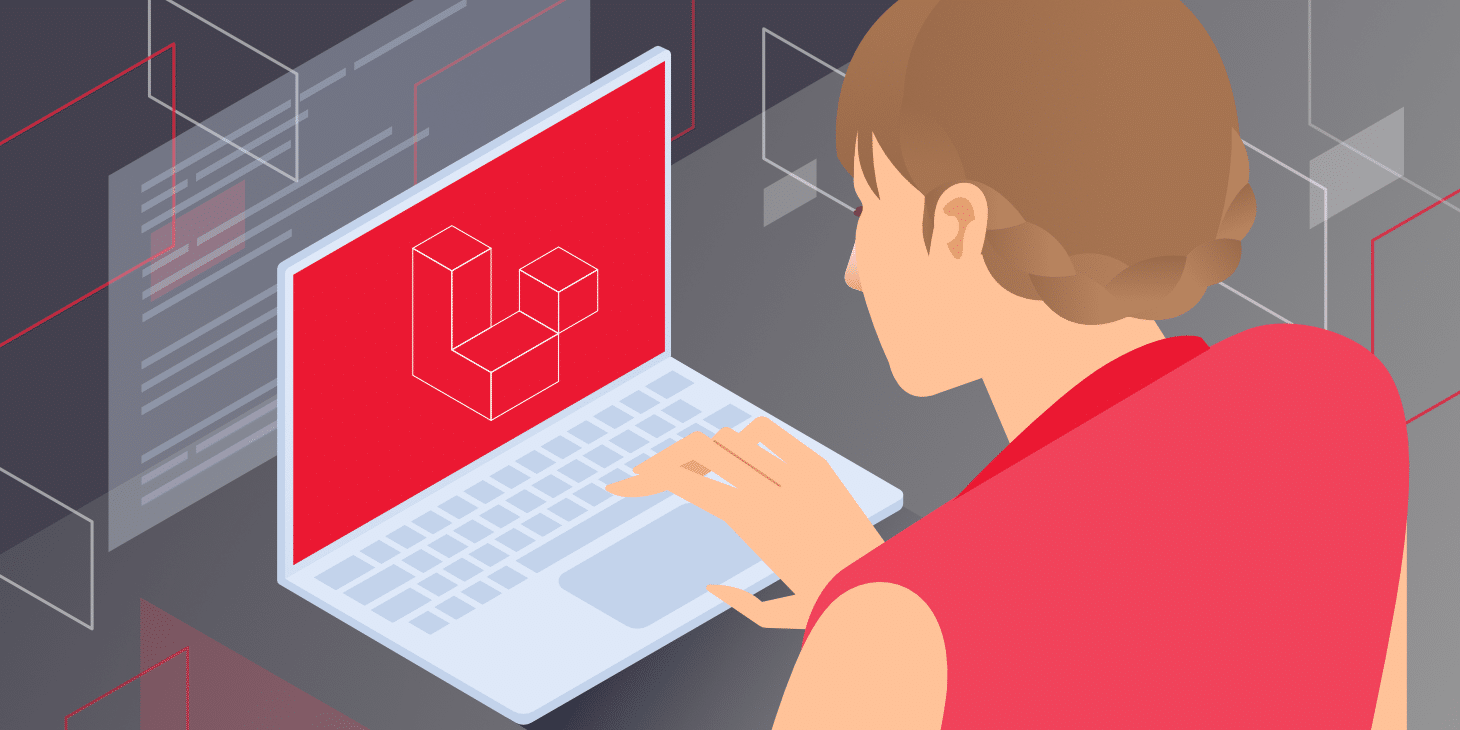
Welcome to the exciting world of Laravel, where scalability meets efficiency! In today’s fast-paced digital landscape, delivering lightning-fast performance is no longer just a luxury; it’s a necessity. That’s where Laravel’s robust caching feature comes into play. With its ability to store frequently accessed data in memory, caching offers a game-changing solution for improving your application’s scalability and overall user experience.
But fear not! Laravel has got you covered with its powerful caching capabilities. By intelligently storing computed results or queried data, you can dramatically reduce the time it takes to serve subsequent requests and ensure smooth sailing even during peak traffic hours.
Ready to dive deeper into the world of Laravel caching? Let’s explore how leveraging this feature can take your application’s scalability to new heights!
Benefits of Caching for Scalability
Caching is an essential tool for improving the scalability of your Laravel application. By storing frequently accessed data in memory, caching reduces the need for repeated database queries or costly computations, resulting in faster response times and improved performance.
One of the key benefits of caching is that it helps alleviate server load during high traffic periods. When multiple users make requests simultaneously, caching ensures that the responses are served from memory instead of executing resource-intensive operations repeatedly. This not only improves overall system efficiency but also allows your application to handle a larger number of concurrent users without experiencing slowdowns or crashes.
Caching can significantly reduce network latency by minimizing external API calls or remote database queries. Instead of making these expensive operations every time a user requests certain data, you can store the results in cache and retrieve them quickly when needed. This not only saves valuable processing time but also minimizes dependencies on external services, making your application more reliable and resilient.
Another advantage of caching is its ability to enhance user experience by delivering content faster. With cached pages or fragments readily available in memory, page load times are drastically reduced. Users will appreciate snappy response times and seamless navigation throughout your application.
Leveraging Laravel’s built-in cache mechanisms enables you to implement advanced features such as rate limiting or throttling with ease. By storing request counts or token-based access rules in cache, you can efficiently control API usage and protect against abuse or unauthorized access attempts.
Incorporating caching into your Laravel application offers numerous benefits for scalability purposes: reducing server load during peak traffic periods; minimizing network latency by avoiding unnecessary resource-intensive operations; improving user experience through faster content delivery; and enabling advanced features like rate limiting and throttling effortlessly.
How to Enable Caching in Laravel
Enabling caching in Laravel is a straightforward process that can greatly enhance the scalability of your application. To enable caching, you need to configure the cache driver in your Laravel configuration file. By default, Laravel uses the file cache driver, which stores cached data in files on your server.
To enable caching, simply open the `config/cache.php` file and set the `default` option to one of the available cache drivers such as “file”, “database”, or “redis”. You can also specify additional options for each driver depending on your specific requirements.
Once caching is enabled, Laravel automatically takes care of storing and retrieving cached data for you. You can use the `Cache` facade provided by Laravel to store values in the cache using simple key-value pairs. Retrieving cached values is just as easy – simply call `Cache::get()` with the corresponding key.
Caching not only improves performance but also reduces database load and network requests. It allows frequently accessed data to be stored temporarily, reducing response times and improving overall user experience.
By leveraging this feature effectively within your application, you can achieve significant improvements in scalability without having to make extensive changes to your codebase. So go ahead and start enabling caching in Laravel today!
Types of Caching Methods Available in Laravel
Laravel provides various caching methods that can be used to improve the performance and scalability of your application. Let’s take a look at some of the types of caching methods available in Laravel.
1. File-based Caching: This method stores cached data in files on disk, making it a simple and easy-to-implement option for small-scale applications. However, it may not be suitable for high-traffic websites as disk I/O can become a bottleneck.
2. Database Caching: With this method, cached data is stored in a database table. It offers better scalability compared to file-based caching as databases are designed to handle large volumes of data efficiently. However, it still involves querying the database, which can impact performance.
3. Memcached/Redis Caching: These methods utilize external key-value stores like Memcached or Redis to store cached data. They offer excellent performance and scalability by keeping the cache in memory rather than on disk or in the database.
4. APCu/OPcache Caching: These PHP extensions provide opcode caching, which improves performance by storing compiled PHP code in memory instead of recompiling it on every request.
Each caching method has its own strengths and weaknesses, so choosing the right one depends on your specific requirements and resources available.
Best Practices for Implementing Caching in Laravel
When it comes to implementing caching in Laravel, there are a few best practices that can help you ensure optimal performance and scalability. First and foremost, it is important to carefully consider what data should be cached. Not all data needs to be cached, so selecting the right items for caching can make a big difference.
Next, it is crucial to establish a clear cache strategy. This involves deciding when and how often the cache should be updated or refreshed. Depending on your application’s requirements, you may need to use different expiration times for different types of data.
Another best practice is to leverage tagging in Laravel’s caching system. By assigning tags to cached items, you can easily invalidate or refresh specific groups of data without affecting others. This provides greater flexibility and control over your cache management.
Take advantage of cache stores such as Redis or Memcached that offer faster retrieval times compared to traditional file-based caching methods. These stores allow for more efficient handling of large amounts of data and improve overall performance.
It is recommended to implement proper error handling when using caching in Laravel. In case there are any issues with retrieving or storing cached items, having appropriate error handling mechanisms in place will help prevent disruptions in your application.
Don’t forget about monitoring and optimizing your cache usage regularly. Keep an eye on cache hit rates and adjust your strategies accordingly based on the actual usage patterns of your application.
By following these best practices for implementing caching in Laravel, you’ll be able to maximize its benefits and enhance the scalability of your application without compromising on performance.
Real-Life Examples of Improved Scalability with Laravel’s Caching
One real-life example where Laravel’s caching feature has greatly improved scalability is in e-commerce websites. These sites often have a high volume of traffic and need to handle numerous requests simultaneously. By implementing caching, the site can store frequently accessed data such as product listings or user information, reducing the load on the database.
Another example is in content-heavy applications such as news websites or blogs. With caching enabled, these sites can generate static versions of pages and serve them to users instead of dynamically generating each request. This significantly reduces server load and improves response times, especially during peak traffic periods.
Laravel’s caching also proves beneficial for APIs that fetch data from external sources. For instance, a weather app that retrieves weather information from an API can cache the results for a certain period so that subsequent requests don’t require unnecessary API calls. This not only speeds up response times but also minimizes dependence on external services.
Application dashboards and reporting systems often deal with complex queries and calculations. By utilizing Laravel’s caching capabilities, these systems can store pre-calculated results temporarily and serve them when requested again. This saves computational resources and enhances overall performance.
Leveraging Laravel’s caching features has proven valuable in various real-life scenarios where scalability is crucial. E-commerce sites experience reduced database load; content-heavy applications benefit from faster page loading times; APIs minimize external service dependencies; and complex reporting systems improve query performance. Incorporating Laravel’s caching functionality enables developers to create efficient and scalable web applications effectively
Common Challenges and Solutions when Using Laravel’s Caching
When using Laravel’s caching feature, there are a few common challenges that developers may face. One challenge is determining the appropriate cache duration for each item. Setting the cache duration too short can result in frequent cache misses and slower performance, while setting it too long can lead to outdated data being served to users.
To overcome this challenge, developers can utilize dynamic caching strategies based on factors such as user activity or data freshness requirements. By implementing a mechanism to invalidate and refresh specific caches when necessary, developers can ensure that users always receive up-to-date information without sacrificing performance.
Another challenge is managing complex relationships between cached items. In some cases, updating one item may require invalidating multiple related caches. This can be particularly challenging in scenarios with deeply nested relationships or intricate dependencies.
To address this challenge, developers can leverage tags provided by Laravel’s caching system. By assigning tags to related cached items and then invalidating all caches associated with a specific tag when an update occurs, developers can efficiently manage complex relationships without manually invalidating each individual cache.
Conclusion
Laravel’s caching feature is a powerful tool that can greatly improve the scalability of your application. By reducing the workload on your database and speeding up response times, caching allows you to handle more traffic and deliver a better user experience.
Enabling caching in Laravel is easy, and there are various methods available to suit different needs. Whether you choose file-based caching, database caching, or an external cache driver like Redis or Memcached, Laravel provides flexibility and options for optimal performance.
Implementing best practices when using Laravel’s caching feature will ensure smooth operation and maximum benefit. Remember to identify the parts of your application that can be cached effectively, set appropriate expiration times for cached data, and regularly monitor cache utilization.
It’s important to be aware of common challenges that may arise when working with Laravel’s caching feature. Issues such as cache invalidation during updates or changes in data structure require careful consideration and planning. By staying informed about potential problems and utilizing appropriate solutions, these challenges can be overcome effectively.
Leveraging Laravel’s Caching feature can significantly enhance the scalability of your application by reducing database load and improving response times. With its flexible options and best practices implementation, you’ll be able to handle increased traffic while providing a seamless user experience.