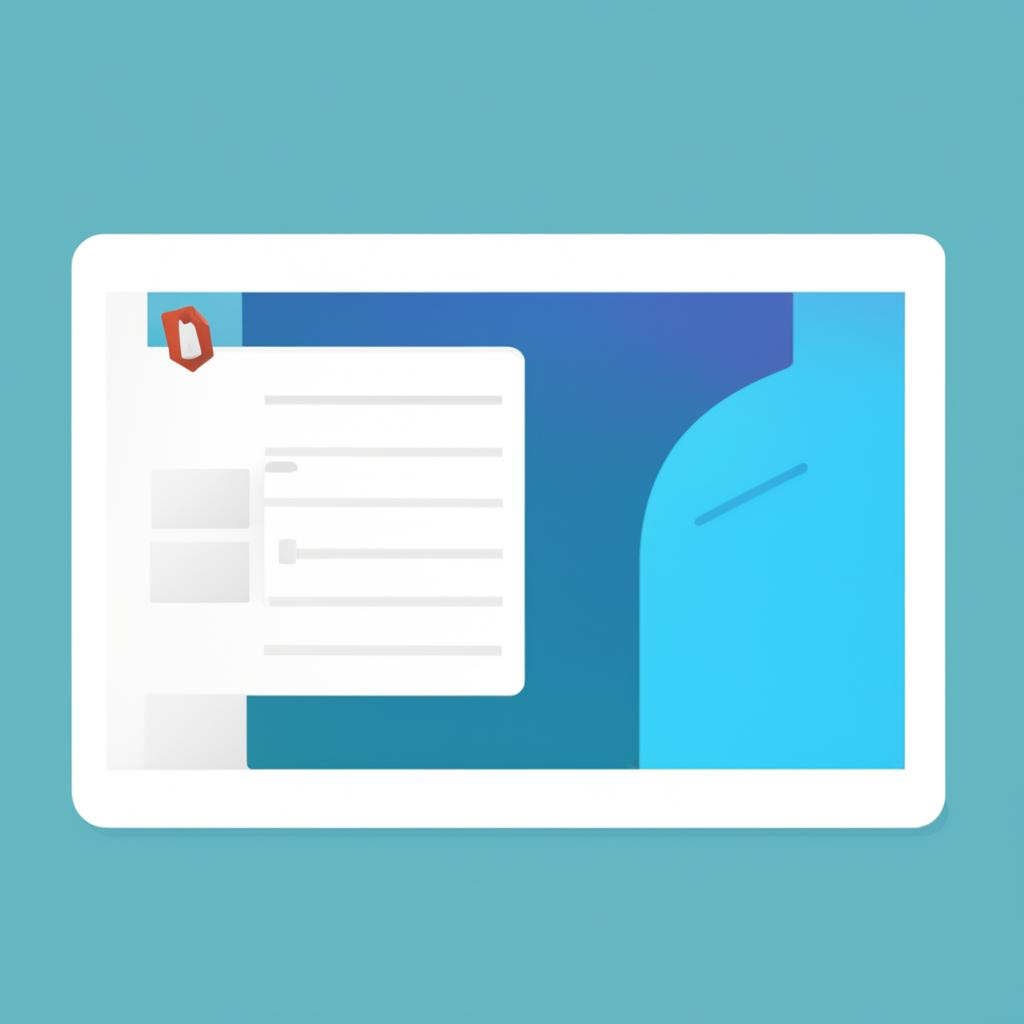
Laravel plays a significant role in automating e-commerce workflows by providing a robust and developer-friendly framework that streamlines the development of scalable and efficient applications. Laravel follows the Model-View-Controller (MVC) architecture, providing an organized structure for building e-commerce applications. The expressive syntax simplifies code readability, making it easier to create and maintain automated workflows.
Laravel’s role in automated e-commerce workflows is substantial, providing developers with a versatile and efficient framework to build applications that meet the demands of modern online retail. Whether it’s managing databases, automating tasks, handling background jobs, or ensuring security, Laravel empowers developers to create robust and scalable e-commerce solutions with streamlined automated workflows.
The Importance of Laravel’s Role in Automated E-commerce Workflows
The importance of Laravel’s role in automated e-commerce workflows lies in its ability to provide a reliable and efficient framework that streamlines development, enhances productivity, and ensures the smooth automation of various tasks within an e-commerce ecosystem. Laravel’s elegant syntax and built-in tools, such as Artisan, significantly speed up development tasks.
Automated e-commerce workflows often involve repetitive processes, and Laravel’s time-saving features contribute to faster project delivery. Laravel’s adherence to the Model-View-Controller (MVC) architecture promotes a well-organized code structure. In automated e-commerce workflows, clarity and organization are crucial, and Laravel’s MVC architecture facilitates efficient development and maintenance.
E-commerce applications heavily rely on database interactions for tasks like order processing, inventory management, and customer data storage. Laravel’s Eloquent ORM simplifies database operations, making it easy to manage and retrieve data, contributing to the automation of various processes.
Laravel’s Artisan command-line tool automates repetitive tasks, including database migrations, seedings, and job scheduling. Automation of routine tasks enhances efficiency, reduces manual errors, and ensures consistency in e-commerce workflows.
Laravel Horizon provides tools for managing and monitoring job queues, crucial for handling background tasks in e-commerce. Asynchronous job processing ensures seamless execution of tasks like order processing and email notifications. Laravel’s middleware allows developers to filter HTTP requests, enabling control over various aspects of the application workflow.
E-commerce workflows often involve complex user interactions, and middleware helps manage authentication, authorization, and other workflow-related tasks. Laravel incorporates built-in security features such as CSRF protection, secure password hashing, and encryption.
Laravel supports robust testing through PHPUnit and Laravel Dusk for browser automation testing. Automated testing ensures the reliability of e-commerce workflows, helping identify and rectify issues early in the development process. Laravel’s extensive ecosystem includes packages that can be seamlessly integrated for specific e-commerce functionalities.
Laravel’s role in automated e-commerce workflows is pivotal for creating scalable, secure, and efficient online retail platforms. Its features, from rapid development tools to robust security measures, contribute to the successful automation of tasks involved in managing and operating e-commerce businesses. Laravel empowers developers to build sophisticated and reliable e-commerce applications, making it a cornerstone in the realm of online retail automation.
The Benefits of using Laravel’s Role in Automated E-commerce Workflows
Laravel’s role in automated e-commerce workflows brings numerous benefits that contribute to the efficiency, reliability, and scalability of online retail operations. Laravel’s expressive syntax and a wealth of built-in features, such as Artisan commands, allow for rapid development of e-commerce workflows.
The MVC architecture in Laravel provides a well-structured codebase, making it easier to maintain, scale, and collaborate on complex e-commerce projects. Quick development cycles are crucial for adapting to changing market demands and implementing new features. Structured code contributes to the longevity and sustainability of the application.
Eloquent simplifies database operations, making it easy to interact with databases and automate tasks like order processing and inventory management. ORM reduces the need for complex SQL queries, enhancing code readability and maintainability.
Artisan automates routine tasks, such as database migrations, seeding, and job scheduling, streamlining the development process. Automation minimizes manual errors and ensures consistency across different environments. Laravel Horizon provides tools for managing job queues efficiently, essential for handling background tasks in e-commerce workflows.
Laravel’s middleware allows developers to filter HTTP requests, enabling fine-grained control over various aspects of the e-commerce workflow. Robust security measures are crucial for maintaining trust and compliance in e-commerce applications. Laravel supports comprehensive testing through PHPUnit and Laravel Dusk for browser automation testing.
Laravel has a large and active community that provides support, resources, and knowledge sharing. Comprehensive documentation ensures that developers have the information needed to work efficiently with Laravel’s features.
Using Laravel’s role in automated e-commerce workflows provides a powerful and versatile foundation for building scalable, secure, and feature-rich online retail applications. The benefits of rapid development, structured code, automated tasks, and a supportive ecosystem make Laravel an ideal choice for creating robust e-commerce platforms that can adapt to the dynamic demands of the online retail landscape.
Step-by-step guide on How to Make Automated E-commerce Workflows with Laravel?
Creating automated e-commerce workflows with Laravel involves a series of steps. Below is a step-by-step guide to help you build an automated e-commerce application using Laravel:
Step 1: Install Laravel
Ensure you have Composer installed. Create a new Laravel project:
composer create-project --prefer-dist laravel/laravel e-commerce-app
Step 2: Set Up Database
Configure your database connection in the .env
file. Run migrations to create necessary tables:
php artisan migrate
Step 3: Create Models
Generate models for essential entities like Product
, Order
, and User
:
php artisan make:model Product -m php artisan make:model Order -m php artisan make:model User -m
Define relationships and fields in migration files.
Step 4: Eloquent Relationships
Define relationships in your models (e.g., Order.php
):
class Order extends Model { public function user() { return $this->belongsTo(User::class); } public function products() { return $this->belongsToMany(Product::class)->withPivot('quantity'); } }
Step 5: Create Controllers
Generate controllers for managing products, orders, and users:
php artisan make:controller ProductController php artisan make:controller OrderController php artisan make:controller UserController
Define actions for CRUD operations in these controllers.
Step 6: Routes
Define routes in routes/web.php
for your controllers:
Route::resource('products', ProductController::class); Route::resource('orders', OrderController::class); Route::resource('users', UserController::class);
Step 7: Views
Create Blade views for displaying products, managing orders, and handling user accounts.
Step 8: Form Requests
Use Form Requests for validating incoming requests:
php artisan make:request CreateOrderRequest
Define validation rules in the request file.
Step 9: Jobs and Queues
Implement jobs for time-consuming tasks. For example, sending order confirmation emails:
php artisan make:job SendOrderConfirmation
Dispatch the job when needed and configure a queue.
Step 10: Event Broadcasting
Implement event broadcasting for real-time updates. For example, broadcasting order status changes.
Step 11: Middleware
Create middleware for tasks like authentication and authorization:
php artisan make:middleware CheckOrderOwnership
Step 12: Testing
Write tests using PHPUnit. Test controllers, models, and features to ensure reliability.
Step 13: Deployment
Deploy your Laravel application to a hosting environment. Configure your web server (e.g., Apache, Nginx).
Step 14: Continuous Integration (Optional)
Implement CI/CD pipelines using tools like GitHub Actions or GitLab CI.
Step 15: Monitoring and Scaling (Optional)
Implement monitoring tools (e.g., Laravel Telescope) and consider strategies for scaling your application.
Following these steps will help you create an automated e-commerce workflow with Laravel. Customize the steps based on your specific requirements and business logic. Laravel’s rich ecosystem, including Eloquent ORM, Artisan commands, and Laravel Mix for asset compilation, simplifies the development process. Adhere to best practices for code organization, security, and performance to ensure a robust and maintainable e-commerce application.
Conclusion
Laravel plays a pivotal role in the development of automated e-commerce workflows, offering a robust and feature-rich framework that empowers developers to build scalable, efficient, and secure online retail applications. Laravel’s elegant syntax and powerful features, including Artisan commands, facilitate rapid development, enabling developers to iterate quickly and adapt to changing market demands.
The adherence to the Model-View-Controller (MVC) architecture ensures a structured and organized codebase, promoting maintainability, scalability, and ease of collaboration among development teams. Eloquent, Laravel’s ORM, simplifies database interactions, making it effortless to manage and automate tasks related to order processing, inventory management, and user data.
Artisan commands automate routine tasks, reducing manual effort and ensuring consistency across different development environments. This automation contributes to increased efficiency in e-commerce workflows. Laravel Horizon provides tools for efficient job processing and queue management, crucial for handling background tasks without affecting the responsiveness of the application. Laravel’s middleware allows fine-grained control over HTTP requests, facilitating tasks such as authentication, authorization, and input validation in the e-commerce workflow.
Built-in security features, including CSRF protection, secure password hashing, and encryption, enhance the overall security of e-commerce applications, safeguarding sensitive customer data. Laravel’s support for comprehensive testing through PHPUnit and Laravel Dusk ensures the reliability of e-commerce workflows by identifying and addressing issues early in the development process.
The Laravel ecosystem includes a variety of packages that can be seamlessly integrated to extend functionality, providing developers with ready-made solutions for common e-commerce features. A vibrant community and comprehensive documentation contribute to the ease of development. Developers can access resources, share knowledge, and seek assistance when building automated e-commerce workflows.
Laravel provides a versatile and developer-friendly environment for creating sophisticated e-commerce applications with streamlined and automated workflows. Its combination of expressive syntax, powerful tools, and a supportive community makes it an excellent choice for developers aiming to build modern, feature-rich, and efficient online retail platforms.