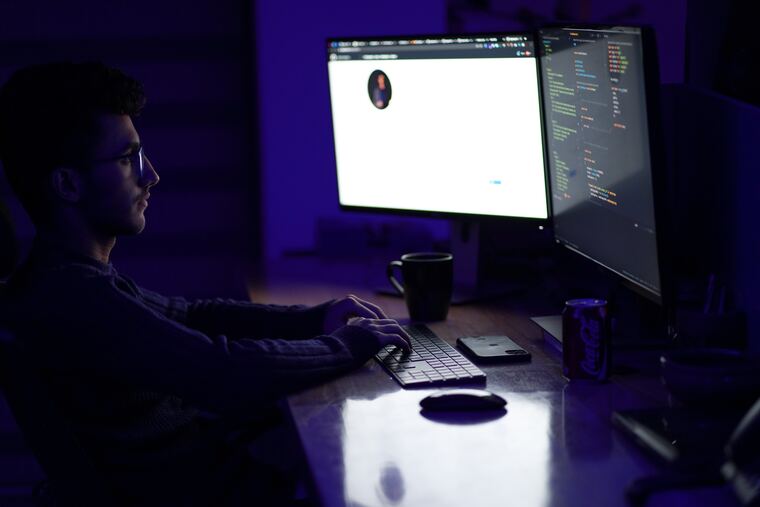
Welcome to the world of Laravel and GraphQL – a dynamic duo that empowers developers to build scalable APIs with ease. If you’re looking for a seamless way to optimize your API development process, then you’ve come to the right place! In this blog post, we’ll dive into the exciting realm of Laravel and GraphQL, exploring their individual strengths and uncovering how they can work together harmoniously.
Laravel is a popular PHP framework renowned for its simplicity and elegance. It provides developers with a solid foundation for building robust web applications swiftly. On the other hand, GraphQL revolutionizes API development by offering flexible querying capabilities, allowing clients to request exactly what they need from an API in a single request.
Combining these two powerhouses not only accelerates development but also enhances performance and scalability. So let’s embark on this journey together as we explore how Laravel and GraphQL can supercharge your API projects!
What is Laravel and Why Use It for API Development?
Laravel, known for its clean and expressive syntax, is a PHP framework that has gained immense popularity among developers. It offers a range of features that make API development a breeze. From routing to database management and authentication, Laravel provides comprehensive solutions for building robust APIs.
One of the main reasons developers choose Laravel for API development is its simplicity. With its intuitive syntax and well-documented codebase, Laravel allows developers to write clean and maintainable code with ease. This means fewer bugs, faster development cycles, and ultimately better productivity.
Another key advantage of using Laravel for API development is its extensive ecosystem. The framework comes bundled with various libraries and tools that streamline common tasks such as database migrations, caching, session management, and more. Additionally, Laravel’s active community ensures that there are numerous packages available to extend the functionality of your APIs effortlessly.
Laravel also excels in providing solid security measures out-of-the-box. With built-in protection against SQL injections and cross-site scripting attacks (XSS), as well as support for user authentication and role-based access control (RBAC), you can rest assured knowing your APIs are secure by default.
Laravel’s ORM (Object Relational Mapping) called Eloquent simplifies working with databases by providing an elegant syntax for querying data without having to write complex SQL statements. This makes it easier to interact with your database tables while maintaining flexibility in defining relationships between models.
Laravel’s simplicity, extensive ecosystem, and strong security measures make it an ideal choice for API development projects.
Understanding GraphQL: Benefits and Implementation
GraphQL is a modern approach to API development that offers several benefits over traditional REST APIs. One of the main advantages of GraphQL is its flexibility in terms of data fetching. Unlike REST, where clients have limited control over the data they receive, GraphQL allows clients to specify exactly what data they need and retrieve it efficiently in a single request.
Another major benefit of GraphQL is its ability to solve the problem of over-fetching and under-fetching data. With REST APIs, developers often struggle with either retrieving too much information or not enough. However, with GraphQL, clients can define their own queries and only request the specific fields they require, eliminating unnecessary network requests and reducing payload size.
GraphQL enables rapid development by allowing frontend teams to work independently from backend teams. This is possible because GraphQL exposes a single endpoint that acts as an interface between clients and servers. Frontend developers can easily fetch the exact data they need without relying on backend changes or creating additional endpoints.
Implementing GraphQL in Laravel is straightforward thanks to libraries like “graphql-php” which provide tools for building schemas and handling query execution within your Laravel application. By defining your schema using type definitions and resolvers, you can map your existing database models to GraphQL types effortlessly.
Laravel’s Eloquent ORM integrates seamlessly with GraphQL making it easy to perform complex database operations through efficient resolver functions. You can also add custom logic such as authentication checks or authorization rules within these resolvers to ensure secure access control for your API.
Understanding the benefits of implementing GraphQL in Laravel opens up exciting possibilities for developing scalable APIs that offer increased flexibility and efficiency compared to traditional REST approaches. Whether you are working on a small project or building large-scale applications,
GraphQL combined with Laravel provides a powerful combination for delivering robust APIs that meet the demands of modern web development.
Setting Up a Basic Laravel Project for GraphQL Integration
Setting up a basic Laravel project for GraphQL integration is an essential step in building scalable APIs. With Laravel’s robust framework and GraphQL’s flexible querying system, developers can create efficient and powerful APIs that meet the demands of modern applications.
To begin, make sure you have Laravel installed on your machine. Open your terminal and navigate to the desired directory where you want to set up your project. Then run the command “composer create-project -prefer-dist laravel/laravel myproject” to install the latest version of Laravel.
Next, install the necessary packages for integrating GraphQL into your Laravel project. Use Composer to require “nuwave/lighthouse” package by running “composer require nuwave/lighthouse”. This package provides tools and utilities that enable seamless GraphQL integration with Laravel.
After installing the required packages, configure your database settings in .env file according to your setup. Then generate a new key for your application by running “php artisan key:generate”.
Now it’s time to define our first GraphQL schema! Create a new folder called “graphql” within app directory and inside it create another folder named “schema”. Within this schema folder, you can start defining your types and queries using SDL (Schema Definition Language).
With all these steps completed, we are now ready to test our basic GraphQL setup in Laravel. Start the development server by executing “php artisan serve” command in terminal. Now open any API testing tool like Insomnia or Postman and send a POST request to http://localhost:8000/graphql endpoint with appropriate query or mutation data.
Creating and Managing GraphQL Schema with Laravel
Creating and managing the GraphQL schema in Laravel is a crucial step in building scalable APIs. The schema defines the structure of your API, including the types of data that can be queried and the operations that can be performed. With Laravel’s built-in support for GraphQL, this process becomes seamless and efficient.
To start, you’ll need to define your schema using the GraphQL SDL (Schema Definition Language). This language allows you to specify the types, queries, mutations, and subscriptions that make up your API. You can easily create new types by extending existing ones or defining custom fields.
Once your schema is defined, you’ll need to map it to corresponding resolvers in Laravel. Resolvers are responsible for fetching data from various sources based on the incoming queries. In Laravel, you can use Eloquent models or query builders as resolvers to retrieve data from databases or external APIs.
Laravel provides powerful tools like GraphiQL IDE for testing your queries and mutations against the defined schema. It offers an intuitive interface where you can explore available types, auto-complete fields, and view documentation about each query or mutation.
Managing your GraphQL schema with Laravel is made easier with tools like Lighthouse – a PHP package specifically designed for implementing GraphQL servers in Laravel applications. Lighthouse simplifies tasks such as validation of input arguments, handling pagination and sorting of results efficiently.
– Defining a well-structured GraphQL schema is essential for building scalable APIs.
– Laravel’s built-in support for GraphQL makes creating and managing schemas effortless.
– Mapping resolvers to their corresponding parts in the schema ensures data retrieval.
– Tools like GraphiQL IDE provide an interactive environment for testing queries.
– Packages like Lighthouse enhance productivity by simplifying common tasks related to implementing GraphQL servers.
Handling Authentication and Authorization in Laravel with GraphQL
Authentication and authorization are crucial aspects of any API development, ensuring that only authorized users can access protected resources. In the context of Laravel and GraphQL, implementing these mechanisms is essential for building secure and robust APIs.
Laravel provides a comprehensive authentication system out of the box, which can be seamlessly integrated with GraphQL. With Laravel’s built-in user authentication features like hashing passwords, managing sessions, and validating credentials, you can easily authenticate users before allowing them to interact with your GraphQL API.
To handle authentication in Laravel with GraphQL, you can leverage middleware to intercept requests and validate access tokens or session data. By defining specific rules based on user roles or permissions within your schema definitions, you can control who has access to certain queries or mutations.
Integrating popular packages like Laravel Passport or JWT (JSON Web Tokens) allows for more advanced authentication methods such as OAuth2 or token-based authentication. These packages provide ready-to-use functionality for generating access tokens, refreshing tokens, and revoking them when necessary.
Authorization is another critical aspect that goes hand-in-hand with authentication. With Laravel’s powerful authorization capabilities using policies and gates, you can define granular permissions at both the application level and individual resource levels.
In combination with GraphQL resolvers—which act as middleware between incoming requests and data sources—you have full control over what data is accessible to each authenticated user based on their role or permissions. This fine-grained control ensures that sensitive information remains protected while still providing a flexible API design.
Handling authentication and authorization in Laravel with GraphQL offers a secure foundation for building scalable APIs. By integrating Laravel’s robust authentication system and leveraging its authorization capabilities, you not only protect your API from unauthorized access but also ensure that every request made through your GraphQL endpoints is handled securely. With proper implementation, you’ll empower developers to create rich applications by utilizing the power of both Laravel and GraphQL.
Performance Considerations for Building Scalable APIs with Laravel and GraphQL
One of the key considerations when building scalable APIs with Laravel and GraphQL is performance. As your API becomes more complex and serves a larger number of requests, it’s important to ensure that it can handle the increased load efficiently.
To optimize performance, you can start by implementing caching mechanisms. Caching allows you to store frequently accessed data in memory or on disk, reducing the time required to fetch and process the data for subsequent requests. Laravel provides built-in support for caching through its caching system, which makes it easy to implement caching strategies in your application.
Another aspect to consider is optimizing database queries. With GraphQL, you have fine-grained control over the data that is requested from your API. By carefully designing your schema and using DataLoader libraries like laravel-graphql-batch or Overblog\GraphQLBundle\BatchResolver\IterablePromiseAdapter, you can minimize unnecessary database queries and consolidate them into efficient batch operations.
Lastly but importantly, regular performance testing should be conducted throughout development and deployment phases. Load testing tools such as Apache JMeter or siege allow you to simulate heavy traffic scenarios on your API endpoints so that any issues related to scalability can be identified early on.
By considering these aspects of performance optimization while building scalable APIs with Laravel and GraphQL, you can ensure that your application delivers fast response times even under high loads.
Real-World Examples of Successful Laravel and GraphQL Implementations
- E-commerce Applications: Many e-commerce platforms have leveraged the power of Laravel and GraphQL to build scalable APIs. By integrating GraphQL with Laravel, these applications can efficiently handle complex queries and provide a flexible data retrieval system for their users.
- Social Networking Platforms: Social media giants like Facebook have implemented GraphQL alongside Laravel to optimize their API performance. With GraphQL’s ability to retrieve only the required data, these platforms can reduce network overhead and enhance user experience by fetching multiple resources in a single request.
- Content Management Systems (CMS): CMS frameworks like WordPress have adopted Laravel with GraphQL integration to offer enhanced customization capabilities for developers. This allows them to create dynamic content models, query specific fields, and make efficient use of caching mechanisms.
- Mobile Application Backends: Mobile app development often requires building robust backends that can handle large amounts of data requests from various clients simultaneously. Combining Laravel with GraphQL enables developers to design highly performant APIs that cater specifically to mobile application needs.
- Data-intensive Analytics Platforms: Companies dealing with massive datasets benefit greatly from using Laravel and GraphQL together due to their ability to process complex queries efficiently. By implementing custom resolvers in the schema, these platforms can aggregate data from multiple sources while maintaining high response times.
These real-world examples demonstrate how organizations across diverse industries have successfully utilized the combination of Laravel and GraphQL for their API development needs.
Challenges and Limitations of Using Laravel and GraphQL Together
While Laravel and GraphQL offer a powerful combination for building scalable APIs, it’s important to be aware of the challenges and limitations that may arise when using them together.
One challenge is the learning curve associated with GraphQL. It requires developers to understand its concepts, such as schema design, querying syntax, and resolver functions. This can take time for those who are new to GraphQL.
Another challenge is the potential complexity of managing relationships between entities in a GraphQL schema. While Laravel provides helpful tools like Eloquent ORM for handling database relationships, mapping these relationships to a GraphQL schema can require additional effort.
Integrating authentication and authorization mechanisms in Laravel with GraphQL can be tricky. Although there are packages available that simplify this process, configuring them correctly may still pose challenges.
Despite some hurdles along the way, combining Laravel’s robustness with the flexibility of GraphQL opens up exciting possibilities for creating scalable APIs that meet modern application demands. With continuous improvement in tooling support and developer expertise growing steadily over time – we can expect even better integration experiences moving forward!