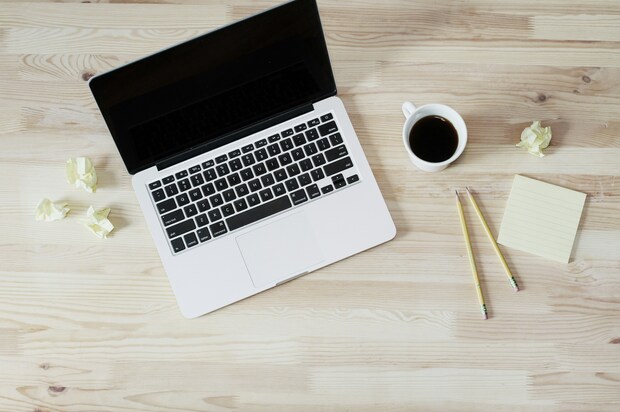
Welcome to the world of secure Laravel APIs! In today’s digital landscape, safeguarding your data is paramount. With cyber threats lurking around every corner, implementing robust security measures is non-negotiable. One such powerful tool in our security arsenal is JSON Web Tokens (JWT). If you’re ready to fortify your Laravel APIs and elevate your data protection game, you’re in the right place! Let’s dive into the realm of JWT and unveil how it can revolutionize the way you secure your APIs.
Understanding Laravel APIs and their Security
Laravel APIs serve as the backbone of many web applications, allowing for seamless communication between the frontend and backend. When it comes to security, protecting these APIs from unauthorized access is paramount. Without proper measures in place, sensitive data can be at risk.
Implementing security features like authentication and authorization ensures that only authenticated users can access protected resources. Laravel provides built-in mechanisms to handle these security concerns effectively.
By understanding how Laravel handles API requests and responses, developers can implement additional layers of security such as JWT (JSON Web Tokens). These tokens provide a secure way to transmit information between parties without compromising sensitive data.
With the right security practices in place, Laravel APIs can operate efficiently while safeguarding against potential threats. It’s crucial for developers to stay informed about best practices and continuously update their knowledge on evolving security trends within the industry.
Step-by-Step Guide: Implementing JWT in Laravel APIs
JWT (JSON Web Token) is a popular method for securing APIs, including those built on Laravel. Implementing JWT in Laravel APIs involves a few key steps to ensure secure authentication and authorization processes.
Start by installing the `tymon/jwt-auth` package using Composer. This package will handle JWT authentication within your Laravel application seamlessly.
Next, configure the JWT secret key in your `.env` file and set up the middleware to authenticate incoming API requests using JWT tokens.
Then, create routes for user login and token refresh endpoints where users can obtain their unique JWT tokens upon successful authentication.
After that, integrate JWT token validation logic in your controllers to restrict access to authenticated routes based on valid tokens.
Don’t forget to handle token expiration and refresh scenarios gracefully to provide a seamless user experience while ensuring security throughout your Laravel API implementation.
Common Challenges and Solutions
When implementing JWT in Laravel APIs, there are common challenges that developers may encounter. One challenge is managing token expiration and refreshing tokens without disrupting the user experience. This can be addressed by setting appropriate token lifetimes and implementing a mechanism to automatically refresh tokens.
Another challenge is securing sensitive data within the JWT payload to prevent unauthorized access. Developers can encrypt sensitive information before encoding it into the token or store sensitive data on the server-side instead of including it in the JWT payload.
Handling token validation and revocation poses a challenge when dealing with compromised tokens or user logout scenarios. Implementing blacklist mechanisms or maintaining a list of revoked tokens can help mitigate this issue effectively.
Ensuring proper error handling for expired or invalid tokens is crucial for providing a seamless user experience. By implementing clear error messages and responses, developers can guide users on how to resolve authentication issues efficiently.
Best Practices for Using JWT in Laravel APIs
When it comes to using JWT in Laravel APIs, there are some best practices that can enhance the security and efficiency of your application. Always ensure to store your JWT secret key securely and never expose it in your codebase. Additionally, regularly rotate your secret keys to mitigate any potential security risks.
Another important practice is to set appropriate expiration times for your JWT tokens to limit their lifespan and reduce the risk of unauthorized access. It’s also recommended to include a payload with relevant information but avoid sensitive data that could compromise security.
Validate incoming JWT tokens thoroughly before granting access to resources within your API endpoints. Implement proper error handling mechanisms for expired or invalid tokens to provide clear feedback to users.
Consider implementing token blacklisting or revocation mechanisms in case a token needs to be invalidated before its expiration time. By following these best practices, you can ensure a more secure and robust authentication process for your Laravel APIs using JWT.
Alternative Authentication Methods for Laravel APIs
Looking to explore alternative authentication methods for your Laravel APIs? One option is OAuth, a robust protocol that allows secure authorization between different systems. With OAuth, users can grant access to their information without sharing passwords directly.
Another approach is API keys, where each user or application is assigned a unique key for accessing the API. This method provides a simple yet effective way to authenticate requests and track usage.
Token-based authentication is also gaining popularity, with tokens generated on the server side and sent back to clients for future requests. This method offers flexibility and scalability in managing user sessions securely.
Integrating social logins like Google or Facebook can streamline the authentication process by leveraging existing accounts. This not only enhances user experience but also reduces registration friction.
Each of these alternative methods comes with its own set of advantages and considerations when implementing authentication in Laravel APIs.
Conclusion
Implementing JWT for secure Laravel APIs is a powerful way to enhance the security of your applications. By understanding how JWT works, following best practices, and being aware of common challenges and solutions, you can effectively protect your APIs from unauthorized access and data breaches.
While there are alternative authentication methods available, JWT stands out as a reliable and widely used option for securing Laravel APIs. Embracing JWT authentication not only provides enhanced security but also allows for seamless integration with various platforms and tools. So, take the necessary steps to implement JWT in your Laravel APIs today and safeguard your applications against potential threats.