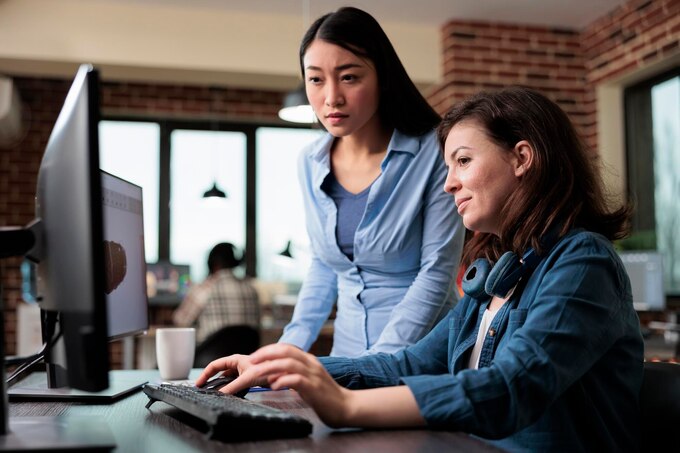
Laravel has taken the web development world by storm. Known for its elegant syntax and powerful features, it allows developers to build robust applications with ease. At the heart of Laravel lies Eloquent ORM (Object-Relational Mapping), a tool that simplifies database interactions while keeping code clean and expressive.
But as your application grows, so do its data needs. That’s where performance optimization becomes crucial. Slow queries can frustrate users and lead to higher bounce rates. Understanding how to enhance Laravel’s performance through efficient Eloquent queries is essential for any developer who wants their application to shine.
In this post, we’ll explore why optimizing Eloquent queries matters, identify common pitfalls, and share valuable techniques that will elevate your application’s speed and efficiency without sacrificing code readability or maintainability. Let’s dive in!
Why Performance Optimization Matters
In today’s fast-paced digital world, users expect applications to be responsive and efficient. Slow-loading pages can lead to frustration and abandonment. Performance optimization is crucial for keeping users engaged.
Moreover, search engines prioritize speed in their ranking algorithms. A well-optimized application can improve your SEO efforts significantly. This means more visibility and potentially higher traffic.
Resource consumption also plays a vital role in performance. Inefficient queries can lead to increased server load, affecting scalability. By optimizing Eloquent queries, you ensure that your application runs smoothly even under heavy traffic.
Additionally, good performance enhances user experience. Happy users are more likely to return and recommend your app to others. Investing time in optimization translates into long-term benefits for both developers and end-users alike.
Common Performance Issues with Eloquent Queries
Eloquent ORM simplifies database interactions, but it can lead to performance pitfalls if not used wisely. One major issue is the “N+1 query problem.” This occurs when your application queries individual records in a loop instead of pulling related data efficiently.
Another common headache is retrieving too much data. Developers often fetch entire models with all their attributes, even when only a few fields are necessary. This can bloat response times and increase memory usage unnecessarily.
Additionally, overly complex queries can slow down performance. Using multiple joins or subqueries without proper indexing can lead to sluggish responses.
Executing frequent database calls during heavy user traffic strains server resources and impacts load times. Addressing these issues upfront saves time and enhances user experience significantly.
Techniques for Optimizing Eloquent Queries
Optimizing Eloquent queries can significantly enhance your Laravel application’s performance. Start by analyzing the queries generated by Eloquent. Use tools like Laravel Debugbar or Telescope to gain insights into query execution time and database calls.
Consider using chunking for large datasets. Instead of loading all records at once, use `chunk()` to process them in smaller batches. This reduces memory usage and speeds up processing times.
Another technique is to limit the fields you retrieve with `select()`. By fetching only necessary columns, you minimize data transfer overhead and improve speed.
Don’t forget about indexing your database tables. Well-placed indexes can drastically reduce search times for commonly queried fields, making lookups much faster.
Keep an eye on N+1 query problems. Using eager loading ensures that related models are loaded efficiently without causing multiple queries per record retrieval.
Utilizing Eager Loading
Eager loading is a powerful technique in Eloquent that helps reduce the number of database queries. By default, Laravel uses lazy loading, which can lead to the N+1 query problem. This occurs when you retrieve a model and then access its related models one by one, resulting in multiple queries.
With eager loading, you can load all related models at once. This means fewer queries overall and improved performance for your application.
To implement eager loading, simply use the `with()` method when retrieving your records. For example, `User::with(‘posts’)->get();` retrieves users along with their posts efficiently.
Not only does this speed up data retrieval, but it also keeps your code cleaner and more readable. Embracing eager loading will elevate your application’s performance while maintaining a smooth user experience.
Using Raw SQL Queries
Raw SQL queries can be a powerful tool for optimizing performance in Laravel. While Eloquent provides an elegant way to interact with your database, sometimes it may not be the best option for complex operations or large datasets.
By using raw SQL, you gain direct control over your database interactions. This often results in faster execution times since you’re bypassing some of the overhead that comes with ORM features.
Laravel allows you to execute raw queries effortlessly through its query builder or by using the DB facade. You can retrieve results as arrays or collections, depending on your needs.
However, it’s crucial to use this method judiciously. Keep an eye on maintainability and readability; overly complex raw queries can lead to confusion later on. Always weigh the benefits against potential drawbacks when opting for this approach.
Conclusion
Enhancing Laravel performance is crucial for building responsive, efficient applications. By understanding Eloquent ORM and its capabilities, developers can unlock the full potential of their projects.
Performance optimization directly impacts user experience. A faster application leads to higher satisfaction and engagement levels. Therefore, it’s essential to identify common pitfalls in Eloquent queries that could slow down your application.
Developers frequently encounter issues like N+1 query problems or excessive database calls. Recognizing these challenges allows for targeted improvements that can significantly boost performance.
Implementing techniques such as eager loading helps minimize database interactions by retrieving related data more efficiently. Utilizing raw SQL queries where appropriate also offers a way to sidestep some limitations of Eloquent while maintaining speed and flexibility.
The path to an optimized Laravel application lies in continually assessing and refining your approach toward query handling within Eloquent. Each enhancement brings you closer to achieving seamless performance tailored for users’ needs.