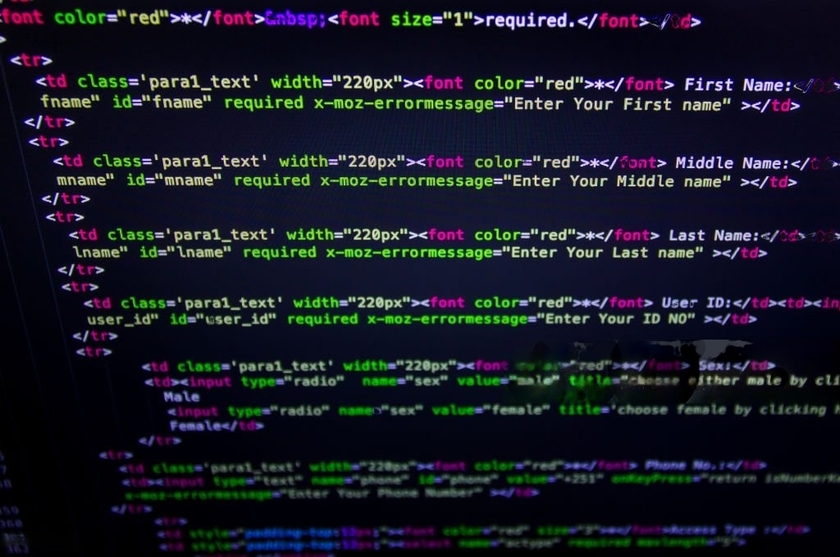
Scaling your application is crucial for handling increased traffic and providing a seamless user experience. As your user base grows, so does the demand on your database and server resources. This is where advanced techniques for scalability come into play, and one tool that can greatly assist in this endeavor is Eloquent, Laravel’s expressive ORM.
We will dive deep into advanced Eloquent techniques that will help you optimize the performance of your application and scale it effectively. From writing efficient queries to utilizing powerful relationships, as well as implementing caching strategies, we’ll cover it all! So grab a cup of coffee and get ready to level up your Laravel skills!
Understanding Scalability and its Importance
Scalability is not just a buzzword; it’s a critical aspect of any application that aspires to grow and handle increased traffic. In simple terms, scalability refers to the ability of your system to maintain its performance even when faced with higher loads.
When your application starts gaining popularity and attracting more users, the demand on your server resources increases exponentially. If you haven’t prepared for this surge in traffic, it can lead to slow response times, downtime, and frustrated users. That’s where scalability comes into play.
By implementing advanced techniques for scalability, you ensure that your application can handle the growing number of requests without compromising performance or user experience. It allows you to seamlessly scale up by adding more servers or upgrading hardware components as needed.
But why is scalability so important? Well, think about it – if your website takes forever to load or crashes frequently under high loads, users will quickly lose interest and move on to a competitor’s site. This directly impacts customer satisfaction and retention rates.
Scaling effectively also helps reduce costs by optimizing resource utilization. When you have an efficiently scalable system in place, you don’t need excess infrastructure sitting idle during low-traffic periods.
Eloquent Models: A Brief Overview
When it comes to building scalable applications, having a solid understanding of Eloquent models is crucial. Laravel’s ORM system, Eloquent, allows you to interact with your database in an intuitive and efficient way.
At its core, an Eloquent model represents a single table within your database. By defining a model for each table, you gain access to powerful features such as querying data, inserting records, updating information, and more.
To create an Eloquent model in Laravel, simply extend the base Model class provided by the framework. This gives you access to all the built-in methods and relationships that make working with databases a breeze.
One of the key benefits of using Eloquent is its expressive syntax. Instead of writing raw SQL queries or dealing with complex joins and subqueries manually, you can leverage Eloquent’s fluent API to build queries easily.
For instance, retrieving all users from the “users” table can be done with just one line of code: `User::all()`. Need only specific columns? No problem! Just use `User::select(‘name’, ’email’)->get()`.
In addition to basic query capabilities, Eloquent also provides support for various advanced techniques like eager loading relationships and utilizing query scopes for reusable query logic. These features help optimize performance by reducing the number of database trips required.
Tips for Writing Efficient Eloquent Queries
When working with Laravel’s Eloquent ORM, writing efficient queries is crucial for improving scalability and overall performance. Here are some tips to help you optimize your code and make the most out of your database interactions.
It’s important to understand that eager loading can significantly enhance query efficiency. By eager loading relationships, you can avoid the N+1 problem where additional queries are executed for each related model. Instead, use the “with” method to load all necessary relationships upfront, reducing unnecessary database hits.
Next, utilize query scopes to encapsulate commonly used query constraints. Scopes allow you to define reusable query logic within your models, making it easier to maintain and modify complex queries as your application grows.
Another tip is to leverage indexing on columns frequently used in search or filtering operations. Adding indexes can greatly improve query execution time by allowing the database engine to quickly locate relevant data.
Consider using raw expressions when performing calculations or aggregations on large datasets. This allows you to bypass Laravel’s automatic quoting of values and write optimized SQL directly in your queries.
Be mindful of the number of records returned in a single request. Limiting result sets using methods like “take” or “paginate” ensures that only essential data is retrieved from the database at any given time.
By following these tips and continuously optimizing your codebase, you’ll be able to write more efficient Eloquent queries that scale well with increasing amounts of data – ultimately improving performance and user experience.
Utilizing Eloquent Relationships for Scalability
When it comes to building scalable applications with Laravel’s Eloquent ORM, understanding and effectively utilizing relationships is key. By defining relationships between your database tables, you can simplify complex queries and improve performance.
One of the most common relationship types in Eloquent is the “belongsTo” relationship. This allows you to define a foreign key on a table that references another table. For example, if you have an “orders” table and a “users” table, you can define a “belongsTo” relationship on the order model to link it back to the user who placed the order.
Another powerful relationship type is the “hasManyThrough” relationship. This allows you to retrieve related records through intermediate tables. It’s especially useful when dealing with deeply nested data structures or when querying large datasets.
Eager loading is another technique that can greatly enhance scalability by reducing query execution time. Rather than making multiple individual queries for related data, eager loading allows you to preload all necessary relationships upfront using the “with()” method. This minimizes database round trips and improves overall performance.
Leveraging polymorphic relationships can help increase flexibility and scalability in your application. Polymorphic relationships allow a model to belong to more than one other model on different tables. This enables dynamic associations without sacrificing simplicity or efficiency.
Don’t forget about pivot tables! Pivot tables are used when working with many-to-many relationships in Eloquent. They provide an efficient way of managing these connections while keeping your codebase clean and maintainable.
By harnessing these various techniques for utilizing eloquent relationships effectively, you’ll be well-equipped to build scalable applications that perform optimally even under heavy loads of data and traffic.
Caching Strategies for Improved Performance
When it comes to improving the performance of your Laravel application, caching is a powerful tool that should not be overlooked. By storing frequently accessed data in cache, you can reduce the number of database queries and speed up your application.
One popular caching strategy is using the built-in Laravel cache system. You can easily store key-value pairs in memory or on disk with just a few lines of code. This allows you to retrieve data quickly without hitting the database every time.
Another technique is leveraging query caching. With this approach, you can cache the results of specific queries and reuse them later if they are requested again within a certain timeframe. This can greatly reduce the load on your database and improve response times.
In addition to these techniques, consider using page caching for static pages or content that doesn’t change frequently. By pre-rendering these pages and saving them as HTML files, you can serve them directly from storage instead of generating them dynamically each time.
By implementing effective caching strategies in your Laravel application, you can significantly improve its performance by reducing database queries and optimizing resource usage. Experiment with different approaches such as Laravel’s built-in cache system, query caching, page caching, and HTTP caching to find what works best for your specific use cases.
Other Advanced Techniques for Scaling with Eloquent
1. Query Optimization: When dealing with large datasets, it’s crucial to optimize your queries for better performance. Take advantage of techniques like eager loading, lazy loading, and selecting only the necessary columns to minimize the query execution time.
2. Indexing Strategies: Properly indexing your database tables can significantly improve query speed and scalability. Identify frequently accessed columns and create indexes on them to reduce the search space and enhance retrieval efficiency.
3. Batch Processing: Instead of processing data one record at a time, consider using batch operations provided by Eloquent. Bulk updates or inserts can drastically reduce the number of database transactions required, resulting in faster execution times.
4. Load Balancing: Distributing incoming requests across multiple servers is a common technique used to handle high traffic loads. Implementing load balancing ensures that each server receives an equal share of the workload, preventing any single server from becoming overwhelmed.
5. Queueing Systems: Offloading resource-intensive tasks to background queues can greatly improve application responsiveness and scalability. Utilize queue systems such as Laravel’s built-in job queues or third-party solutions like Redis or RabbitMQ for efficient task handling.
6. Database Replication: Replicating your database allows you to distribute read operations across multiple servers while maintaining a single source of truth for write operations. This can help alleviate read bottlenecks by parallelizing read requests across replicas.
7. Caching Layers: Integrate caching layers into your application architecture to store frequently accessed data in memory rather than querying it from the database repeatedly.
Conclusion
We’ve explored some advanced techniques for using Eloquent models to achieve scalability in your Laravel applications. We began by understanding the importance of scalability and how it can impact the performance and user experience of your application.
We then delved into a brief overview of Eloquent models, highlighting their power and flexibility in working with databases. From there, we provided valuable tips for writing efficient Eloquent queries, ensuring that you can retrieve data quickly and effectively.
We discussed how to leverage Eloquent relationships to improve scalability. By establishing connections between different models, you can efficiently retrieve related data without resorting to multiple database queries.
We also explored caching strategies as a means of enhancing performance. By temporarily storing frequently accessed data in memory or on disk, you can significantly reduce the load on your database server and deliver faster responses to users.
We touched upon other advanced techniques for scaling with Eloquent. These include optimizing indexing strategies for large datasets and utilizing eager loading to minimize lazy-loading overhead.
Remember that achieving scalability is an ongoing process that requires continuous monitoring and refinement. Regularly analyzing performance metrics will help identify bottlenecks so they can be addressed promptly.